坦克大战c++ windows.h
时间: 2023-09-27 15:05:29 浏览: 40
坦克大战是一款经典的游戏,可以用C++和Windows.h库来实现。下面是一个简单的实现示例:
```cpp
#include <iostream>
#include <Windows.h>
using namespace std;
const int MAP_WIDTH = 20;
const int MAP_HEIGHT = 20;
const int TANK_SIZE = 3;
const int BULLET_SIZE = 1;
char g_map[MAP_HEIGHT][MAP_WIDTH];
class Tank
{
public:
Tank(int x, int y, char direction)
: m_x(x), m_y(y), m_direction(direction)
{
for (int i = 0; i < TANK_SIZE; i++)
{
g_map[y][x + i] = 'T';
}
}
void move(char direction)
{
switch (direction)
{
case 'w':
if (m_y > 0 && g_map[m_y - 1][m_x] == ' ')
{
clear();
m_y--;
draw();
}
break;
case 's':
if (m_y < MAP_HEIGHT - 1 && g_map[m_y + TANK_SIZE][m_x] == ' ')
{
clear();
m_y++;
draw();
}
break;
case 'a':
if (m_x > 0 && g_map[m_y][m_x - 1] == ' ')
{
clear();
m_x--;
draw();
}
break;
case 'd':
if (m_x < MAP_WIDTH - TANK_SIZE && g_map[m_y][m_x + TANK_SIZE] == ' ')
{
clear();
m_x++;
draw();
}
break;
default:
break;
}
m_direction = direction;
}
void fire()
{
Bullet bullet(m_x + TANK_SIZE / 2, m_y + TANK_SIZE / 2, m_direction);
}
private:
void draw()
{
for (int i = 0; i < TANK_SIZE; i++)
{
g_map[m_y][m_x + i] = 'T';
}
}
void clear()
{
for (int i = 0; i < TANK_SIZE; i++)
{
g_map[m_y][m_x + i] = ' ';
}
}
int m_x;
int m_y;
char m_direction;
};
class Bullet
{
public:
Bullet(int x, int y, char direction)
: m_x(x), m_y(y), m_direction(direction)
{
g_map[y][x] = 'B';
}
void move()
{
switch (m_direction)
{
case 'w':
if (m_y > 0 && g_map[m_y - 1][m_x] == ' ')
{
clear();
m_y--;
draw();
}
else
{
clear();
}
break;
case 's':
if (m_y < MAP_HEIGHT - 1 && g_map[m_y + 1][m_x] == ' ')
{
clear();
m_y++;
draw();
}
else
{
clear();
}
break;
case 'a':
if (m_x > 0 && g_map[m_y][m_x - 1] == ' ')
{
clear();
m_x--;
draw();
}
else
{
clear();
}
break;
case 'd':
if (m_x < MAP_WIDTH - 1 && g_map[m_y][m_x + 1] == ' ')
{
clear();
m_x++;
draw();
}
else
{
clear();
}
break;
default:
break;
}
}
private:
void draw()
{
g_map[m_y][m_x] = 'B';
}
void clear()
{
g_map[m_y][m_x] = ' ';
}
int m_x;
int m_y;
char m_direction;
};
void initMap()
{
for (int i = 0; i < MAP_HEIGHT; i++)
{
for (int j = 0; j < MAP_WIDTH; j++)
{
if (i == 0 || i == MAP_HEIGHT - 1 || j == 0 || j == MAP_WIDTH - 1)
{
g_map[i][j] = '#';
}
else
{
g_map[i][j] = ' ';
}
}
}
}
void printMap()
{
system("cls");
for (int i = 0; i < MAP_HEIGHT; i++)
{
for (int j = 0; j < MAP_WIDTH; j++)
{
cout << g_map[i][j];
}
cout << endl;
}
}
int main()
{
initMap();
Tank tank(1, 1, 'd');
while (true)
{
printMap();
char input = getchar();
if (input == ' ')
{
tank.fire();
}
else
{
tank.move(input);
}
Sleep(100);
}
return 0;
}
```
这是一个简单的示例,你可以根据需要进行修改和完善。
相关推荐
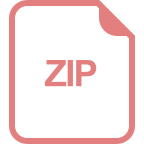
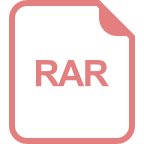














