安卓(Android studio)编写一个计算器,可以连续加减乘除,可加小数点,不包含括号以及加减乘除优先算法。、
时间: 2023-06-15 22:06:15 浏览: 57
好的,以下是一个简单的安卓计算器的示例代码。我们使用一个TextView来显示输入和结果,以及一些Button来输入数字和操作符。在代码中,我们使用一个StringBuilder对象来保存输入的内容,并在操作符按钮被点击时将其添加到TextView中。最后,我们在等号按钮被点击时计算表达式并将结果显示在TextView中。
MainActivity.java:
```
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
private TextView inputTextView;
private StringBuilder inputStringBuilder;
private boolean lastButtonWasOperator = false;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
inputTextView = findViewById(R.id.inputTextView);
inputStringBuilder = new StringBuilder();
// Set up number buttons
for (int i = 0; i <= 9; i++) {
int id = getResources().getIdentifier("button" + i, "id", getPackageName());
Button button = findViewById(id);
button.setOnClickListener(this);
}
// Set up operator buttons
Button plusButton = findViewById(R.id.buttonPlus);
plusButton.setOnClickListener(this);
Button minusButton = findViewById(R.id.buttonMinus);
minusButton.setOnClickListener(this);
Button multiplyButton = findViewById(R.id.buttonMultiply);
multiplyButton.setOnClickListener(this);
Button divideButton = findViewById(R.id.buttonDivide);
divideButton.setOnClickListener(this);
Button decimalButton = findViewById(R.id.buttonDecimal);
decimalButton.setOnClickListener(this);
Button clearButton = findViewById(R.id.buttonClear);
clearButton.setOnClickListener(this);
Button equalsButton = findViewById(R.id.buttonEquals);
equalsButton.setOnClickListener(this);
}
@Override
public void onClick(View view) {
switch (view.getId()) {
case R.id.button0:
addInput("0");
break;
case R.id.button1:
addInput("1");
break;
case R.id.button2:
addInput("2");
break;
case R.id.button3:
addInput("3");
break;
case R.id.button4:
addInput("4");
break;
case R.id.button5:
addInput("5");
break;
case R.id.button6:
addInput("6");
break;
case R.id.button7:
addInput("7");
break;
case R.id.button8:
addInput("8");
break;
case R.id.button9:
addInput("9");
break;
case R.id.buttonPlus:
addOperator("+");
break;
case R.id.buttonMinus:
addOperator("-");
break;
case R.id.buttonMultiply:
addOperator("*");
break;
case R.id.buttonDivide:
addOperator("/");
break;
case R.id.buttonDecimal:
addInput(".");
break;
case R.id.buttonClear:
clearInput();
break;
case R.id.buttonEquals:
calculate();
break;
}
}
private void addInput(String input) {
inputStringBuilder.append(input);
inputTextView.setText(inputStringBuilder.toString());
lastButtonWasOperator = false;
}
private void addOperator(String operator) {
if (!lastButtonWasOperator) {
inputStringBuilder.append(operator);
inputTextView.setText(inputStringBuilder.toString());
lastButtonWasOperator = true;
}
}
private void clearInput() {
inputStringBuilder = new StringBuilder();
inputTextView.setText("");
lastButtonWasOperator = false;
}
private void calculate() {
String input = inputStringBuilder.toString();
String[] parts = input.split("[+\\-*/]");
double result = Double.parseDouble(parts[0]);
int operatorIndex = 0;
for (int i = 1; i < parts.length; i++) {
String operator = input.substring(operatorIndex + parts[i - 1].length(), operatorIndex + parts[i - 1].length() + 1);
double operand = Double.parseDouble(parts[i]);
switch (operator) {
case "+":
result += operand;
break;
case "-":
result -= operand;
break;
case "*":
result *= operand;
break;
case "/":
result /= operand;
break;
}
operatorIndex += parts[i - 1].length() + 1;
}
inputTextView.setText(Double.toString(result));
inputStringBuilder = new StringBuilder(Double.toString(result));
lastButtonWasOperator = false;
}
}
```
activity_main.xml:
```
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/inputTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="right"
android:textSize="24sp"
android:textStyle="bold"
android:padding="8dp"
android:textColor="@android:color/black"
android:background="@android:color/white"/>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<Button
android:id="@+id/button7"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="7"/>
<Button
android:id="@+id/button8"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="8"/>
<Button
android:id="@+id/button9"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="9"/>
<Button
android:id="@+id/buttonDivide"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="/"/>
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<Button
android:id="@+id/button4"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="4"/>
<Button
android:id="@+id/button5"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="5"/>
<Button
android:id="@+id/button6"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="6"/>
<Button
android:id="@+id/buttonMultiply"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="*"/>
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<Button
android:id="@+id/button1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="1"/>
<Button
android:id="@+id/button2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="2"/>
<Button
android:id="@+id/button3"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="3"/>
<Button
android:id="@+id/buttonMinus"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="-"/>
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<Button
android:id="@+id/button0"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="0"/>
<Button
android:id="@+id/buttonDecimal"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="."/>
<Button
android:id="@+id/buttonClear"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="C"/>
<Button
android:id="@+id/buttonPlus"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="+"/>
</LinearLayout>
<Button
android:id="@+id/buttonEquals"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="="/>
</LinearLayout>
```
相关推荐
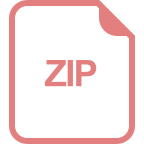














