安卓(Android studio)编写一个计算器,可以连续加减乘除,可加小数点,不包含括号以及加减乘除优先算法
时间: 2023-06-14 16:08:07 浏览: 73
好的,以下是一个简单的安卓计算器代码示例:
1. 在 XML 文件中创建布局:
```
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/resultTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="30sp"
android:textAlignment="center"/>
<LinearLayout
android:layout_below="@id/resultTextView"
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:weightSum="4">
<Button
android:id="@+id/button1"
android:text="1"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/button2"
android:text="2"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/button3"
android:text="3"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/buttonAdd"
android:text="+"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
</LinearLayout>
<LinearLayout
android:layout_below="@id/button1"
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:weightSum="4">
<Button
android:id="@+id/button4"
android:text="4"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/button5"
android:text="5"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/button6"
android:text="6"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/buttonSubtract"
android:text="-"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
</LinearLayout>
<LinearLayout
android:layout_below="@id/button4"
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:weightSum="4">
<Button
android:id="@+id/button7"
android:text="7"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/button8"
android:text="8"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/button9"
android:text="9"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/buttonMultiply"
android:text="*"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
</LinearLayout>
<LinearLayout
android:layout_below="@id/button7"
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:weightSum="4">
<Button
android:id="@+id/buttonClear"
android:text="C"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/button0"
android:text="0"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/buttonDecimal"
android:text="."
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
<Button
android:id="@+id/buttonDivide"
android:text="/"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content" />
</LinearLayout>
<Button
android:id="@+id/buttonEqual"
android:text="="
android:layout_below="@id/buttonClear"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</RelativeLayout>
```
2. 在 Java 文件中添加以下代码:
```
public class MainActivity extends AppCompatActivity {
private TextView resultTextView;
private String operand1 = "";
private String operand2 = "";
private String operator = "";
private String result = "";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
resultTextView = findViewById(R.id.resultTextView);
// Set up number buttons
findViewById(R.id.button0).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
numberPressed("0");
}
});
findViewById(R.id.button1).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
numberPressed("1");
}
});
findViewById(R.id.button2).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
numberPressed("2");
}
});
findViewById(R.id.button3).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
numberPressed("3");
}
});
findViewById(R.id.button4).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
numberPressed("4");
}
});
findViewById(R.id.button5).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
numberPressed("5");
}
});
findViewById(R.id.button6).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
numberPressed("6");
}
});
findViewById(R.id.button7).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
numberPressed("7");
}
});
findViewById(R.id.button8).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
numberPressed("8");
}
});
findViewById(R.id.button9).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
numberPressed("9");
}
});
findViewById(R.id.buttonDecimal).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
numberPressed(".");
}
});
// Set up operator buttons
findViewById(R.id.buttonAdd).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
operatorPressed("+");
}
});
findViewById(R.id.buttonSubtract).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
operatorPressed("-");
}
});
findViewById(R.id.buttonMultiply).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
operatorPressed("*");
}
});
findViewById(R.id.buttonDivide).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
operatorPressed("/");
}
});
// Set up clear button
findViewById(R.id.buttonClear).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
clearPressed();
}
});
// Set up equal button
findViewById(R.id.buttonEqual).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
equalPressed();
}
});
}
private void numberPressed(String number) {
if (operator.isEmpty()) {
operand1 += number;
resultTextView.setText(operand1);
} else {
operand2 += number;
resultTextView.setText(operand2);
}
}
private void operatorPressed(String operator) {
this.operator = operator;
resultTextView.setText(operator);
}
private void clearPressed() {
operand1 = "";
operand2 = "";
operator = "";
result = "";
resultTextView.setText("0");
}
private void equalPressed() {
if (!operand1.isEmpty() && !operand2.isEmpty() && !operator.isEmpty()) {
double num1 = Double.parseDouble(operand1);
double num2 = Double.parseDouble(operand2);
switch (operator) {
case "+":
result = String.valueOf(num1 + num2);
break;
case "-":
result = String.valueOf(num1 - num2);
break;
case "*":
result = String.valueOf(num1 * num2);
break;
case "/":
if (num2 == 0) {
result = "Error";
} else {
result = String.valueOf(num1 / num2);
}
break;
}
resultTextView.setText(result);
operand1 = result;
operand2 = "";
operator = "";
result = "";
}
}
}
```
这样就可以实现一个简单的安卓计算器了。
相关推荐
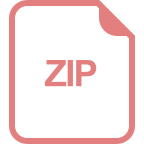














