实现顺序栈和链栈的基本操作,包括创建栈、入栈、出栈、读取栈顶元素等操作并在主函数中测试各操作
时间: 2023-12-21 13:03:24 浏览: 104
顺序栈基本操作实现:
```c++
#include <iostream>
using namespace std;
const int MAXSIZE = 100;
class SeqStack {
public:
SeqStack() {
top = -1;
}
bool isEmpty() {
return top == -1;
}
bool isFull() {
return top == MAXSIZE - 1;
}
bool push(int x) {
if (isFull()) {
return false;
}
data[++top] = x;
return true;
}
bool pop() {
if (isEmpty()) {
return false;
}
--top;
return true;
}
int topElem() {
if (isEmpty()) {
return -1;
}
return data[top];
}
private:
int data[MAXSIZE];
int top;
};
int main() {
SeqStack s;
// push
for (int i = 0; i < 10; ++i) {
s.push(i);
}
// topElem
cout << "top: " << s.topElem() << endl;
// pop
while (!s.isEmpty()) {
cout << s.topElem() << " ";
s.pop();
}
cout << endl;
return 0;
}
```
链栈基本操作实现:
```c++
#include <iostream>
using namespace std;
class LinkStack {
public:
LinkStack() {
top = nullptr;
}
bool isEmpty() {
return top == nullptr;
}
bool push(int x) {
Node *newNode = new Node(x);
newNode->next = top;
top = newNode;
return true;
}
bool pop() {
if (isEmpty()) {
return false;
}
Node *tmp = top->next;
delete top;
top = tmp;
return true;
}
int topElem() {
if (isEmpty()) {
return -1;
}
return top->data;
}
private:
class Node {
public:
Node(int x) {
data = x;
next = nullptr;
}
int data;
Node *next;
};
Node *top;
};
int main() {
LinkStack s;
// push
for (int i = 0; i < 10; ++i) {
s.push(i);
}
// topElem
cout << "top: " << s.topElem() << endl;
// pop
while (!s.isEmpty()) {
cout << s.topElem() << " ";
s.pop();
}
cout << endl;
return 0;
}
```
阅读全文
相关推荐

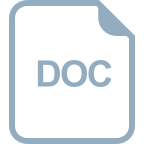
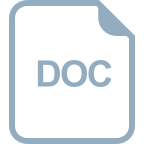












