用c语言写一个彩票项目
时间: 2023-11-23 08:06:53 浏览: 204
一个完整的彩票项目涉及到很多方面,包括彩票购买、开奖、中奖等等。下面是一个简单的彩票项目,用于模拟用户购买彩票、开奖以及判断用户是否中奖。
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 彩票信息
typedef struct {
int nums[7]; // 7个号码
int price; // 价格
} Lottery;
// 随机生成一个彩票
Lottery generateLottery() {
Lottery lottery;
int i;
srand(time(NULL)); // 使用系统时间作为随机数种子
for (i = 0; i < 7; i++) {
lottery.nums[i] = rand() % 10; // 生成 0~9 的随机数
}
lottery.price = 2; // 彩票价格为 2 元
return lottery;
}
// 购买彩票
void buyLottery(Lottery *lotteries, int count) {
int i;
printf("欢迎购买彩票,每张彩票价格为 2 元。\n");
for (i = 0; i < count; i++) {
lotteries[i] = generateLottery();
printf("第 %d 张彩票已生成,号码为:", i + 1);
for (int j = 0; j < 7; j++) {
printf("%d ", lotteries[i].nums[j]);
}
printf("\n");
}
printf("购买成功,总共购买了 %d 张彩票,共需支付 %d 元。\n", count, count * 2);
}
// 开奖
Lottery drawLottery() {
Lottery lottery = generateLottery();
printf("本期彩票已开奖,号码为:");
for (int j = 0; j < 7; j++) {
printf("%d ", lottery.nums[j]);
}
printf("\n");
return lottery;
}
// 判断是否中奖
void checkLottery(Lottery *lotteries, int count, Lottery lottery) {
int i, j;
int matchCount = 0;
printf("开始判断是否中奖...\n");
for (i = 0; i < count; i++) {
matchCount = 0;
for (j = 0; j < 7; j++) {
if (lotteries[i].nums[j] == lottery.nums[j]) {
matchCount++;
}
}
if (matchCount >= 3) { // 中奖条件为至少猜对 3 个号码
printf("第 %d 张彩票中奖了,中了 %d 个号码,获得了 %d 元奖金!\n", i + 1, matchCount, matchCount * 5);
} else {
printf("第 %d 张彩票未中奖!\n", i + 1);
}
}
}
int main() {
Lottery lotteries[10];
int count;
printf("请输入要购买的彩票数量:");
scanf("%d", &count);
buyLottery(lotteries, count);
Lottery lottery = drawLottery();
checkLottery(lotteries, count, lottery);
return 0;
}
```
运行程序,按照提示输入要购买的彩票数量,程序会自动生成相应数量的彩票号码,并计算出总共需要支付的金额。然后进行开奖,并判断用户是否中奖,最后输出中奖信息和奖金。这只是一个简单的模拟,实际的彩票项目还需要考虑更多的因素。
阅读全文
相关推荐
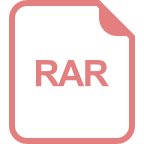
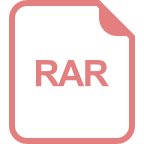
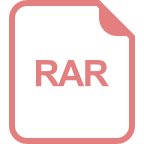
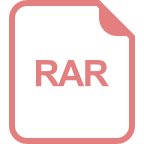
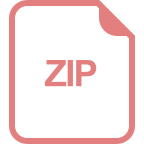
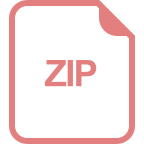
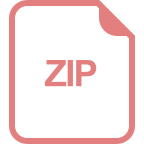
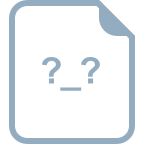
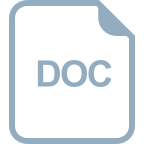
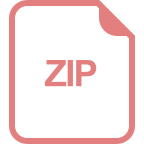
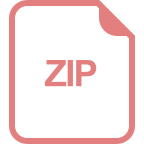
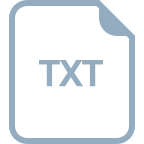
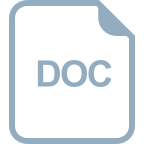
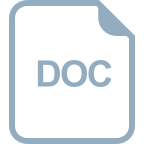
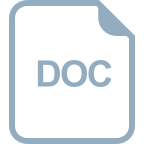
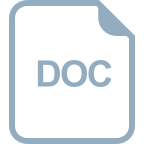
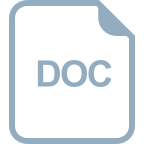