time_pattern = re.compile(r'(\d{4})-(\d{2})-(\d{2})T(\d{2}):(\d{2}):(\d{2})\.(\d{3})')
时间: 2024-01-19 19:06:23 浏览: 128
这是一个 Python 中使用 re 模块定义的正则表达式,用于匹配符合特定格式的时间字符串。具体来说,它可以匹配形如 "2022-01-01T12:34:56.789" 的时间字符串,其中年、月、日、小时、分钟、秒和毫秒分别用数字表示,且用 "T" 和 "." 分隔不同的时间部分。正则表达式中使用了括号来将年、月、日、小时、分钟、秒和毫秒分别分组,方便后续使用。
相关问题
import requests import os import time import json from tqdm import tqdm import re def taopiaopiao(): headers = { 'user-agent': 'Mozilla/5.0 (Linux; Android 6.0; Nexus 5 Build/MRA58N) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/113.0.0.0 Mobile Safari/537.36 Edg/113.0.1774.57' } time.sleep(0.5) url = "https://dianying.taobao.com/showList.htm?spm=a1z21.6646273.city.2.4ed46d6ekOc3wH&n_s=new&city=310100" response = requests.get(url, headers=headers) html = response.text print("网页信息已获取…") time.sleep(0.5) destinationPath = "result.txt" fd = open(destinationPath, "w+", encoding='utf-8') fd.writelines(html) end = html.find('<!-- 即将热映 -->') if end != -1: html = html[:end] #print(html) fd.close() s = '<img width="160" height="224" data-src="(.*?)" src=' + \ '.*?<span class="bt-l">(.+?)</span>.*?<span class="bt-r">(\d.\d)?</span>' + \ ".*?<span>导演:(.*?)</span>" + ".*?<span>主演:(.*?)</span>" + ".*?<span>类型:(.*?)</span>" + \ ".*?<span>地区:(.*?)</span>" + ".*?<span>语言:(.*?)</span>" + ".*?<span>片长:(.*?)</span>" + \ ".*?<a href=(.*?)>" pattern = re.compile(s, re.S) items = re.findall(pattern, html) #print(items) destinationPath = "items.json" fd = open(destinationPath, "w+", encoding='utf-8') json.dump(items, fd) fd.close() dir_name = "./images" if not os.path.exists(dir_name): os.mkdir(dir_name) cnt = 0 for item in tqdm(items): url = item[0] file_name = str(cnt) + ".jpg" cnt += 1 response = requests.get(url, headers=headers) with open(dir_name + "/" + file_name, 'wb') as f: f.write(response.content) info = "图片文件: {0:25}{1}".format(file_name, " 成功下载...") print(info) return items if __name__ == "__main__": taopiaopiao()
这段代码存在一个问题,就是在导入requests模块时没有使用正确的语法。应该在每个导入模块的语句之间添加一个空格。请尝试修改import语句,如下所示:
```
import requests
import os
import time
import json
from tqdm import tqdm
import re
```
这样修改后,代码应该就可以正常运行了。如果还有其他问题,请告诉我。
Python re.compile
re.compile() is a function in Python's built-in re (regular expression) module that compiles a regular expression pattern into a regular expression object. The syntax for using re.compile() is:
```
re.compile(pattern, flags=0)
```
where:
- `pattern`: the regular expression pattern to be compiled.
- `flags`: optional flags that modify the behavior of the regular expression matching. It can be a combination of the following flags:
- `re.IGNORECASE` or `re.I`: case-insensitive matching.
- `re.MULTILINE` or `re.M`: multiline matching.
- `re.DOTALL` or `re.S`: dot matches all, including newlines.
- `re.VERBOSE` or `re.X`: verbose mode, allowing whitespace and comments in the pattern.
The re.compile() function returns a compiled regular expression object that can be used for matching against strings with the re module's search(), match(), findall(), and other functions. By compiling the pattern ahead of time, you can reuse the compiled object in multiple matching operations, which can improve performance.
阅读全文
相关推荐
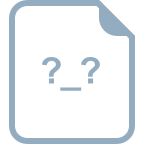
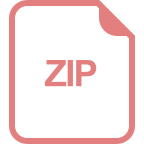


用正则表达式.*?board-index.*?>(.*?).*?data-src="(.*?)".*?name.*?a.*?>(.*?)
.*?star.*?>(.*?).*?releasetime.*?>(.*?).*?integer.*?>(.*?).*?fraction.*?>(.*?).*?爬取猫眼电影排行:爬取猫眼电影TOP100的所有信息。网址:https://www.maoyan.com/board/4 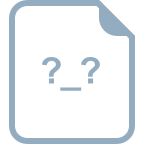
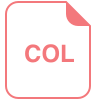
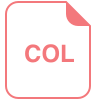
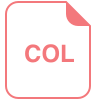
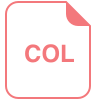
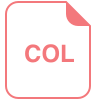
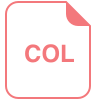
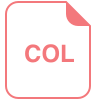
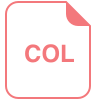
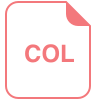
