用python修改json文件后怎么写入并保存
时间: 2023-05-11 20:07:00 浏览: 181
你可以使用Python内置的json库来读取和修改json文件。首先,你需要使用`json.load()`函数来读取json文件并将其转换为Python对象。然后,你可以修改这个Python对象,最后使用`json.dump()`函数将修改后的Python对象写入到json文件中。
以下是一个示例代码:
```python
import json
# 读取json文件
with open('example.json', 'r') as f:
data = json.load(f)
# 修改Python对象
data['name'] = 'Alice'
# 将修改后的Python对象写入json文件
with open('example.json', 'w') as f:
json.dump(data, f)
```
在这个示例中,我们首先使用`json.load()`函数读取了名为`example.json`的json文件,并将其转换为Python对象`data`。然后,我们修改了`data`对象中的`name`属性。最后,我们使用`json.dump()`函数将修改后的`data`对象写入到`example.json`文件中。
注意,如果你想要格式化输出json文件,可以在`json.dump()`函数中添加`indent`参数,例如:
```python
json.dump(data, f, indent=4)
```
这将使输出的json文件具有缩进,更易于阅读。
相关问题
python输出json文件保存
你可以使用Python中的`json`模块来实现将数据保存为JSON文件。以下是一个简单的示例代码:
```python
import json
data = {
"name": "John",
"age": 30,
"city": "New York"
}
# 将数据保存为JSON文件
with open('data.json', 'w') as f:
json.dump(data, f)
```
在上述示例中,我们先定义了一个字典`data`,后使用`json.dump()`函数将数据写入名为`data.json`的文件中。你可以根据需要修改文件名和文件路径。
python读取json文件并且修改里面内容后,在将文件保存
在Python中,你可以使用`json`模块来读取JSON文件,操作数据后再将其保存回文件。这里是一个简单的步骤示例:
1. **打开并读取JSON文件**:
```python
import json
# 打开文件
with open('example.json', 'r') as file:
# 加载 JSON 数据
data = json.load(file)
```
2. **修改文件内容**:
假设你想修改某个键值对,比如把`"key"`的值从`old_value`改为`new_value`:
```python
data["key"] = "new_value"
```
3. **保存修改后的JSON文件**:
```python
# 写入文件
with open('example.json', 'w') as file:
# 将修改后的字典转换回JSON格式并写入
json.dump(data, file, ensure_ascii=False) # `ensure_ascii=False` 避免中文乱码
```
如果你需要替换整个数据结构而不是单个键值对,可以直接赋给新的变量,然后替换原文件:
```python
new_data = {
# ... 新的数据结构 ...
}
with open('example.json', 'w') as file:
json.dump(new_data, file, ensure_ascii=False)
```
阅读全文
相关推荐
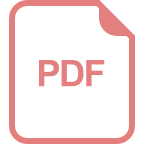
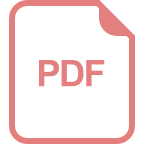
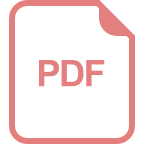
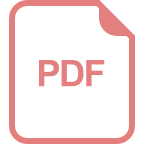









