java实现模糊搜索查询功能
时间: 2023-08-08 09:12:45 浏览: 196
好的,实现模糊搜索查询功能可以使用SQL语句中的LIKE操作符,配合通配符%或_使用。具体的实现步骤如下:
1. 获取用户输入的搜索关键字。
2. 构建SQL语句,使用LIKE操作符和通配符%来实现模糊搜索。例如,如果用户输入的关键字为“apple”,则可以使用如下的SQL语句进行搜索:
SELECT * FROM products WHERE name LIKE '%apple%';
其中,products为要搜索的表名,name为要搜索的字段名,%apple%即为搜索的关键字。
3. 执行SQL语句,获取查询结果。
4. 将查询结果返回给用户。
下面是一个简单的Java代码示例,用于实现模糊搜索查询功能:
```
public List<Product> searchProducts(String keyword) {
Connection conn = null;
PreparedStatement stmt = null;
ResultSet rs = null;
List<Product> result = new ArrayList<>();
try {
// 连接数据库
conn = getConnection();
// 构建SQL语句
String sql = "SELECT * FROM products WHERE name LIKE ?";
// 创建PreparedStatement对象
stmt = conn.prepareStatement(sql);
// 设置参数
stmt.setString(1, "%" + keyword + "%");
// 执行查询
rs = stmt.executeQuery();
// 遍历结果集
while (rs.next()) {
Product product = new Product();
product.setId(rs.getInt("id"));
product.setName(rs.getString("name"));
product.setPrice(rs.getDouble("price"));
// 将查询结果添加到列表中
result.add(product);
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 关闭连接
close(conn, stmt, rs);
}
return result;
}
```
在上述代码中,getConnection()方法用于获取数据库连接,close()方法用于关闭连接和释放资源。Product是一个JavaBean,用于表示查询结果的数据结构。
相关推荐
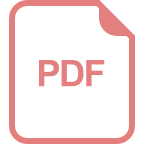
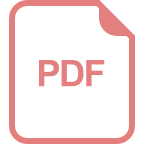
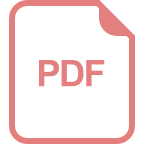














