springboot+mybatisplus+vue实现模糊查询
时间: 2023-07-14 18:14:04 浏览: 146
首先,在前端页面上添加一个输入框用于输入搜索关键字,并且在点击搜索按钮时将关键字传递到后端。
然后,在后端的控制器中,接收前端传递的关键字参数,并且调用MyBatisPlus提供的模糊查询API进行查询。
具体实现步骤如下:
1.前端页面实现
在前端页面上添加一个输入框和搜索按钮,当用户在输入框输入了关键字并点击搜索按钮时,将关键字传递到后端。
```vue
<template>
<div>
<input v-model="keyword" />
<button @click="search">搜索</button>
</div>
</template>
<script>
export default {
data() {
return {
keyword: '',
};
},
methods: {
search() {
this.$axios.get('/api/search', { params: { keyword: this.keyword } }).then((res) => {
console.log(res.data);
});
},
},
};
</script>
```
2.后端控制器实现
在后端控制器中,接收前端传递的关键字参数,并且调用MyBatisPlus提供的模糊查询API进行查询。这里我们使用MyBatisPlus提供的QueryWrapper对象来构建查询条件,并且使用Lambda表达式来简化代码。
```java
@RestController
@RequestMapping("/api")
public class SearchController {
@Autowired
private UserService userService;
@GetMapping("/search")
public List<User> search(String keyword) {
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.like("name", keyword).or().like("email", keyword);
return userService.list(wrapper);
}
}
```
在上面的代码中,我们构建了一个QueryWrapper对象,使用like方法来查询name或者email字段中包含关键字的数据。最终调用userService的list方法来执行查询操作。
需要注意的是,在这里我们假设UserService是一个MyBatisPlus提供的服务类,并且已经配置好了MapperScannerConfigurer来扫描Mapper接口。如果还没有配置,可以参考MyBatisPlus的文档进行配置。
3.配置MyBatisPlus
在使用MyBatisPlus的模糊查询功能前,需要先配置MyBatisPlus。在SpringBoot中,只需要在配置文件中添加如下配置即可:
```yaml
mybatis-plus:
mapper-locations: classpath:/mapper/**/*.xml
global-config:
db-config:
logic-delete-value: 1
logic-not-delete-value: 0
configuration:
map-underscore-to-camel-case: true
```
在上面的配置中,我们指定了Mapper接口XML文件的位置,配置了逻辑删除的值和驼峰命名规则等。
4.编写Mapper接口和XML文件
最后,我们需要编写Mapper接口和XML文件来实现具体的查询操作。这里我们以User表为例,编写如下代码:
```java
public interface UserMapper extends BaseMapper<User> {
}
```
```xml
<mapper namespace="com.example.mapper.UserMapper">
<resultMap id="BaseResultMap" type="com.example.entity.User">
<id column="id" property="id" />
<result column="name" property="name" />
<result column="email" property="email" />
</resultMap>
<select id="selectByKeyword" resultMap="BaseResultMap">
select * from user
where name like concat('%', #{keyword}, '%')
or email like concat('%', #{keyword}, '%')
</select>
</mapper>
```
在上面的代码中,我们使用了MyBatisPlus提供的BaseMapper接口,并且编写了一个selectByKeyword方法来执行模糊查询操作。需要注意的是,我们使用了concat函数来将%和关键字拼接起来,以实现模糊查询的效果。
至此,我们已经完成了springboot+mybatisplus+vue实现模糊查询的全部操作。
相关推荐
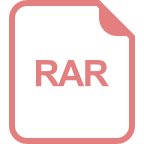
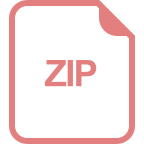














