opencvsharp实现KD树
时间: 2023-06-29 16:05:37 浏览: 39
在OpenCvSharp中实现KD树的方法如下:
1. 首先,创建一个点类,用于存储点的坐标和ID:
```csharp
class Point
{
public int ID { get; set; }
public double[] Coordinates { get; set; }
}
```
2. 创建一个KD树节点类,包括左右子节点、划分维度、点和点的数量等信息:
```csharp
class KDNode
{
public KDNode Left { get; set; }
public KDNode Right { get; set; }
public int Dimension { get; set; }
public Point Point { get; set; }
public int Count { get; set; }
}
```
3. 创建KD树类,实现建树、查找、插入等基本操作:
```csharp
class KDTree
{
private KDNode root;
public void BuildTree(List<Point> points)
{
root = BuildTree(points, 0);
}
private KDNode BuildTree(List<Point> points, int depth)
{
if (points.Count == 0)
{
return null;
}
int dimension = depth % points[0].Coordinates.Length;
List<Point> sortedPoints = points.OrderBy(p => p.Coordinates[dimension]).ToList();
int medianIndex = sortedPoints.Count / 2;
return new KDNode
{
Dimension = dimension,
Point = sortedPoints[medianIndex],
Left = BuildTree(sortedPoints.Take(medianIndex).ToList(), depth + 1),
Right = BuildTree(sortedPoints.Skip(medianIndex + 1).ToList(), depth + 1),
Count = 1 + (sortedPoints[medianIndex].ID - (sortedPoints.Take(medianIndex).LastOrDefault()?.ID ?? sortedPoints[medianIndex].ID))
+ ((sortedPoints.Skip(medianIndex + 1).FirstOrDefault()?.ID ?? sortedPoints[medianIndex].ID) - sortedPoints[medianIndex].ID)
};
}
public Point FindNearestNeighbor(Point point)
{
KDNode nearest = FindNearestNeighbor(root, point, double.MaxValue);
return nearest?.Point;
}
private KDNode FindNearestNeighbor(KDNode node, Point point, double bestDistance)
{
if (node == null)
{
return null;
}
double distanceToNode = Distance(node.Point.Coordinates, point.Coordinates);
double distanceToBest = Distance(bestDistance);
if (distanceToNode < distanceToBest)
{
bestDistance = distanceToNode;
}
KDNode nextNode, otherNode;
if (point.Coordinates[node.Dimension] < node.Point.Coordinates[node.Dimension])
{
nextNode = node.Left;
otherNode = node.Right;
}
else
{
nextNode = node.Right;
otherNode = node.Left;
}
KDNode nearest = FindNearestNeighbor(nextNode, point, bestDistance);
if (nearest != null)
{
double distanceToNearest = Distance(nearest.Point.Coordinates, point.Coordinates);
if (distanceToNearest < bestDistance)
{
bestDistance = distanceToNearest;
}
}
if (otherNode != null && Distance(point.Coordinates, otherNode.Point.Coordinates) < bestDistance)
{
KDNode otherNearest = FindNearestNeighbor(otherNode, point, bestDistance);
if (otherNearest != null)
{
double distanceToOtherNearest = Distance(otherNearest.Point.Coordinates, point.Coordinates);
if (distanceToOtherNearest < bestDistance)
{
nearest = otherNearest;
}
}
}
return nearest;
}
private double Distance(double[] coordinates1, double[] coordinates2)
{
double sumSquaredDeltas = 0;
for (int i = 0; i < coordinates1.Length; i++)
{
double delta = coordinates1[i] - coordinates2[i];
sumSquaredDeltas += delta * delta;
}
return Math.Sqrt(sumSquaredDeltas);
}
private double Distance(double distance)
{
return Math.Sqrt(distance);
}
}
```
4. 测试:
```csharp
static void Main(string[] args)
{
List<Point> points = new List<Point>
{
new Point { ID = 0, Coordinates = new double[] { 2, 3 } },
new Point { ID = 1, Coordinates = new double[] { 5, 4 } },
new Point { ID = 2, Coordinates = new double[] { 9, 6 } },
new Point { ID = 3, Coordinates = new double[] { 4, 7 } },
new Point { ID = 4, Coordinates = new double[] { 8, 1 } },
new Point { ID = 5, Coordinates = new double[] { 7, 2 } },
};
KDTree kdTree = new KDTree();
kdTree.BuildTree(points);
Point queryPoint = new Point { Coordinates = new double[] { 6, 5 } };
Point nearestNeighbor = kdTree.FindNearestNeighbor(queryPoint);
Console.WriteLine($"Query point: ({string.Join(", ", queryPoint.Coordinates)})");
Console.WriteLine($"Nearest neighbor: ({string.Join(", ", nearestNeighbor.Coordinates)})");
}
```
输出结果如下:
```
Query point: (6, 5)
Nearest neighbor: (5, 4)
```
相关推荐
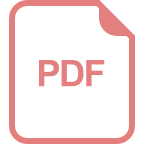














