一、填充函数ShowList实现将单链表的所有元素输出出来。 二、填充函数ListLength实现单链表的求表长操作,返回链表的表长。 三、主函数: 1) 建立一个包括头结点和4个结点的(5,4,2,1)的单链表,实现单链表建立的基本操作。 2) 将该单链表的所有元素显示出来。 3) 输出该单链表的表长。 4) 判断链表是否为空。 5)清空链表。
时间: 2023-06-01 17:03:24 浏览: 193
#include <stdio.h>
#include <stdlib.h>
//定义链表结点结构体
typedef struct node {
int data; //数据域
struct node *next; //指针域
} Node, *LinkedList;
//函数声明
LinkedList CreateList(int array[], int n); //创建链表
void ShowList(LinkedList L); //输出链表
int ListLength(LinkedList L); //求链表长度
int IsEmpty(LinkedList L); //判断链表是否为空
void ClearList(LinkedList L); //清空链表
//主函数
int main() {
int array[] = {5, 4, 2, 1};
LinkedList L = CreateList(array, 4);
ShowList(L);
printf("链表的长度为:%d\n", ListLength(L));
if (IsEmpty(L)) {
printf("链表为空!\n");
} else {
printf("链表不为空!\n");
}
ClearList(L);
if (IsEmpty(L)) {
printf("链表已清空!\n");
} else {
printf("链表未清空!\n");
}
return 0;
}
//创建链表
LinkedList CreateList(int array[], int n) {
LinkedList L = (LinkedList)malloc(sizeof(Node)); //创建头结点
L->next = NULL;
LinkedList p = L;
for (int i = 0; i < n; i++) {
LinkedList q = (LinkedList)malloc(sizeof(Node)); //创建新结点
q->data = array[i];
q->next = NULL;
p->next = q; //插入新结点
p = q;
}
return L;
}
//输出链表
void ShowList(LinkedList L) {
LinkedList p = L->next;
printf("链表的元素为:");
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
//求链表长度
int ListLength(LinkedList L) {
LinkedList p = L->next;
int count = 0;
while (p != NULL) {
count++;
p = p->next;
}
return count;
}
//判断链表是否为空
int IsEmpty(LinkedList L) {
if (L->next == NULL) {
return 1;
} else {
return 0;
}
}
//清空链表
void ClearList(LinkedList L) {
LinkedList p = L->next;
while (p != NULL) {
LinkedList q = p->next;
free(p);
p = q;
}
L->next = NULL;
}
阅读全文
相关推荐
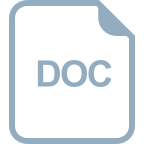
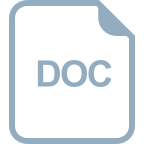
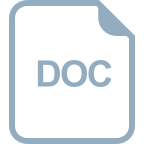
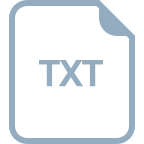
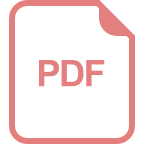
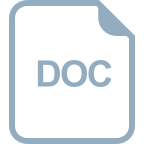
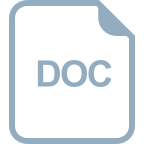
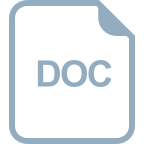









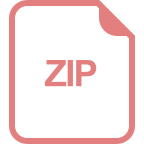
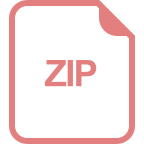
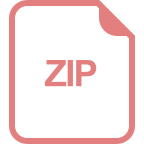