给我一个俄罗斯方块的c语言代码
时间: 2024-05-09 11:15:59 浏览: 73
以下是一个简单的俄罗斯方块游戏的 C 语言代码。
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <conio.h>
#define ROW 20
#define COL 10
int board[ROW][COL] = {0};
int curBlock[4][4] = {0};
int curX = 0, curY = 0;
int score = 0;
void init();
void draw();
void genBlock();
int checkBlock(int x, int y);
int checkLine(int line);
int checkGameOver();
void clearLine(int line);
void moveDown();
void moveLeft();
void moveRight();
void rotate();
void updateBoard();
void clearBoard();
int main() {
init();
while(1) {
draw();
if(checkGameOver()) {
printf("Game Over! Your score is %d.", score);
break;
}
if(kbhit()) {
int ch = getch();
switch(ch) {
case 'a':
moveLeft();
break;
case 'd':
moveRight();
break;
case 's':
moveDown();
break;
case 'w':
rotate();
break;
}
}
else {
moveDown();
}
}
return 0;
}
void init() {
srand(time(NULL));
genBlock();
}
void draw() {
system("cls");
printf("Score: %d\n", score);
for(int i = 0; i < ROW; i++) {
for(int j = 0; j < COL; j++) {
if(board[i][j] == 1) {
printf("* ");
}
else {
printf(". ");
}
}
printf("\n");
}
}
void genBlock() {
int blocks[7][4][4] = {
{{1, 1, 0, 0}, {1, 1, 0, 0}, {0, 0, 0, 0}, {0, 0, 0, 0}}, // square
{{1, 1, 0, 0}, {0, 1, 1, 0}, {0, 0, 0, 0}, {0, 0, 0, 0}}, // S
{{0, 1, 1, 0}, {1, 1, 0, 0}, {0, 0, 0, 0}, {0, 0, 0, 0}}, // Z
{{1, 1, 1, 0}, {0, 1, 0, 0}, {0, 0, 0, 0}, {0, 0, 0, 0}}, // L
{{1, 1, 1, 0}, {1, 0, 0, 0}, {0, 0, 0, 0}, {0, 0, 0, 0}}, // J
{{0, 1, 0, 0}, {1, 1, 1, 0}, {0, 0, 0, 0}, {0, 0, 0, 0}}, // T
{{0, 1, 0, 0}, {0, 1, 0, 0}, {0, 1, 0, 0}, {0, 1, 0, 0}} // I
};
int r = rand() % 7;
for(int i = 0; i < 4; i++) {
for(int j = 0; j < 4; j++) {
curBlock[i][j] = blocks[r][i][j];
}
}
curX = 3;
curY = 0;
}
int checkBlock(int x, int y) {
for(int i = 0; i < 4; i++) {
for(int j = 0; j < 4; j++) {
if(curBlock[i][j]) {
if(x + j < 0 || x + j >= COL || y + i >= ROW) {
return 0;
}
if(y + i >= 0 && board[y+i][x+j]) {
return 0;
}
}
}
}
return 1;
}
int checkLine(int line) {
for(int j = 0; j < COL; j++) {
if(board[line][j] == 0) {
return 0;
}
}
return 1;
}
int checkGameOver() {
return !checkBlock(curX, curY+1);
}
void clearLine(int line) {
for(int i = line; i > 0; i--) {
for(int j = 0; j < COL; j++) {
board[i][j] = board[i-1][j];
}
}
score += 100;
}
void moveDown() {
if(checkBlock(curX, curY+1)) {
curY++;
}
else {
updateBoard();
}
}
void moveLeft() {
if(checkBlock(curX-1, curY)) {
curX--;
}
}
void moveRight() {
if(checkBlock(curX+1, curY)) {
curX++;
}
}
void rotate() {
int tmp[4][4];
for(int i = 0; i < 4; i++) {
for(int j = 0; j < 4; j++) {
tmp[i][j] = curBlock[3-j][i];
}
}
for(int i = 0; i < 4; i++) {
for(int j = 0; j < 4; j++) {
curBlock[i][j] = tmp[i][j];
}
}
if(!checkBlock(curX, curY)) {
for(int i = 1; i < 4; i++) {
if(checkBlock(curX-i, curY)) {
curX -= i;
break;
}
if(checkBlock(curX+i, curY)) {
curX += i;
break;
}
}
for(int i = 1; i < 4; i++) {
if(checkBlock(curX, curY-i)) {
curY -= i;
break;
}
}
}
}
void updateBoard() {
for(int i = 0; i < 4; i++) {
for(int j = 0; j < 4; j++) {
if(curBlock[i][j]) {
board[curY+i][curX+j] = 1;
}
}
}
for(int i = ROW-1; i >= 0; i--) {
if(checkLine(i)) {
clearLine(i);
i++;
}
}
genBlock();
}
void clearBoard() {
for(int i = 0; i < ROW; i++) {
for(int j = 0; j < COL; j++) {
board[i][j] = 0;
}
}
}
```
请注意,此代码可能需要在 Windows 平台上编译和运行,因为它使用了 `conio.h` 头文件中的 `kbhit()` 和 `getch()` 函数,这些函数在 Unix/Linux 系统上可能不可用。
阅读全文
相关推荐





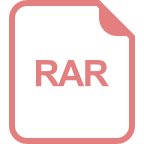

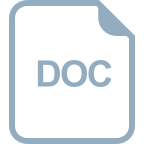






