解释一下下面一段代码 def detect(self, name_list, img): showimg = img with torch.no_grad(): img = letterbox(img, new_shape=self.opt.img_size)[0] img = img[:, :, ::-1].transpose(2, 0, 1) # BGR to RGB, to 3x416x416 img = np.ascontiguousarray(img) img = torch.from_numpy(img).to(self.device) img = img.half() if self.half else img.float() # uint8 to fp16/32 img /= 255.0 # 0 - 255 to 0.0 - 1.0 if img.ndimension() == 3: img = img.unsqueeze(0) pred = self.model(img, augment=self.opt.augment)[0] pred = non_max_suppression(pred, self.opt.conf_thres, self.opt.iou_thres, classes=self.opt.classes, agnostic=self.opt.agnostic_nms) info_show = "" for i, det in enumerate(pred): if det is not None and len(det): det[:, :4] = scale_coords(img.shape[2:], det[:, :4], showimg.shape).round() for *xyxy, conf, cls in reversed(det): label = '%s %.2f' % (self.names[int(cls)], conf) name_list.append(self.names[int(cls)]) single_info = plot_one_box2(xyxy, showimg, label=label, color=self.colors[int(cls)], line_thickness=2) # print(single_info) info_show = info_show + single_info + "\n" return info_show
时间: 2023-12-04 10:06:27 浏览: 121
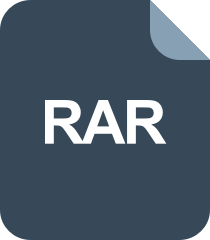
lirc_serial.rar_Space Between
这段代码是一个函数 detect,接受两个参数 name_list 和 img,其中 name_list 是一个列表,img 是一个图像。这个函数的作用是对图像进行目标检测,返回一个字符串 info_show。
在函数内部,首先将原始图像 showimg 赋值给变量 showimg。然后使用 letterbox 函数调整图像大小到指定大小(self.opt.img_size),并进行一些预处理操作,将其转换为 PyTorch 的张量格式。接着使用模型 self.model 对图像进行预测,得到预测结果 pred。
对于每个预测结果 det,将其框的坐标进行还原,然后使用 plot_one_box2 函数在图像上绘制出矩形框和标签,并将标签信息添加到 name_list 中。最终将所有标签信息合并为一个字符串 info_show 并返回。
阅读全文
相关推荐
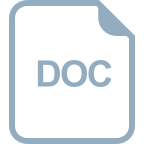
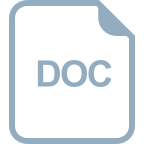


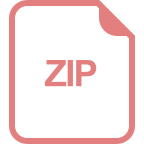
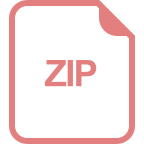
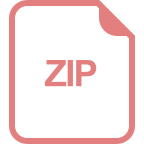
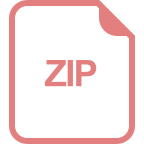
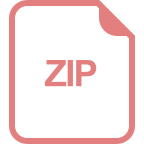
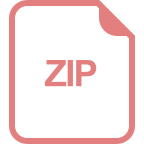
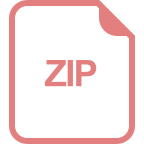
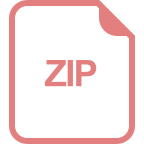
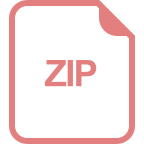
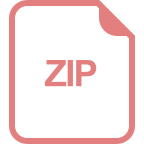
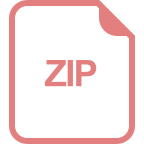
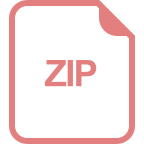
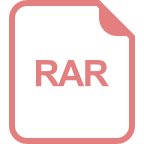
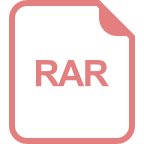