解释一下下面一段代码 def detect(self, name_list, img): showimg = img with torch.no_grad(): img = letterbox(img, new_shape=self.opt.img_size)[0] img = img[:, :, ::-1].transpose(2, 0, 1) # BGR to RGB, to 3x416x416 img = np.ascontiguousarray(img) img = torch.from_numpy(img).to(self.device) img = img.half() if self.half else img.float() # uint8 to fp16/32 img /= 255.0 # 0 - 255 to 0.0 - 1.0 if img.ndimension() == 3: img = img.unsqueeze(0) pred = self.model(img, augment=self.opt.augment)[0] pred = non_max_suppression(pred, self.opt.conf_thres, self.opt.iou_thres, classes=self.opt.classes, agnostic=self.opt.agnostic_nms) info_show = "" for i, det in enumerate(pred): if det is not None and len(det): det[:, :4] = scale_coords(img.shape[2:], det[:, :4], showimg.shape).round() for *xyxy, conf, cls in reversed(det): label = '%s %.2f' % (self.names[int(cls)], conf) name_list.append(self.names[int(cls)]) single_info = plot_one_box2(xyxy, showimg, label=label, color=self.colors[int(cls)], line_thickness=2) # print(single_info) info_show = info_show + single_info + "\n" return info_show
时间: 2023-12-04 20:06:27 浏览: 42
这是一个函数,名称为 detect,其输入参数有 name_list 和 img,其中 name_list 是一个列表,img 是一个图片。该函数的作用是使用 YOLOv5 检测算法对输入的图片进行目标检测,并返回检测结果的字符串形式。
具体实现过程如下:
1. 将输入的 img 赋值给 showimg 变量。
2. 使用 letterbox 函数将 img 调整为指定大小(416x416)。
3. 将 BGR 图像转换为 RGB 并转置通道顺序,同时将数据类型转换为 numpy 数组。
4. 将 numpy 数组转换为 PyTorch 张量,并将数据类型转换为 float32 或 float16(如果设置了 self.half,则为 float16)。
5. 将像素值从 0-255 映射到 0.0-1.0。
6. 如果输入的张量维度为 3,则在第 0 维添加一个维度。
7. 使用 YOLOv5 模型对输入的张量进行预测,得到预测结果 pred。
8. 对预测结果进行非极大值抑制(NMS)处理,得到去除冗余框后的预测结果。
9. 遍历预测结果,对每个预测框进行绘制和标注,并将检测到的物体类别名称添加到 name_list 列表中。
10. 将检测结果的字符串形式返回。
相关问题
解释一下下面一段代码 def detect(self, name_list, img): showimg = img with torch.no_grad(): img = letterbox(img, new_shape=self.opt.img_size)[0] img = img[:, :, ::-1].transpose(2, 0, 1) # BGR to RGB, to 3x416x416 img = np.ascontiguousarray(img) img = torch.from_numpy(img).to(self.device) img = img.half() if self.half else img.float() # uint8 to fp16/32 img /= 255.0 # 0 - 255 to 0.0 - 1.0 if img.ndimension() == 3: img = img.unsqueeze(0) pred = self.model(img, augment=self.opt.augment)[0] pred = non_max_suppression(pred, self.opt.conf_thres, self.opt.iou_thres, classes=self.opt.classes, agnostic=self.opt.agnostic_nms) info_show = "" for i, det in enumerate(pred): if det is not None and len(det): det[:, :4] = scale_coords(img.shape[2:], det[:, :4], showimg.shape).round() for *xyxy, conf, cls in reversed(det): label = '%s %.2f' % (self.names[int(cls)], conf) name_list.append(self.names[int(cls)]) single_info = plot_one_box2(xyxy, showimg, label=label, color=self.colors[int(cls)], line_thickness=2) # print(single_info) info_show = info_show + single_info + "\n" return info_show
这段代码是一个函数 detect,接受两个参数 name_list 和 img,其中 name_list 是一个列表,img 是一个图像。这个函数的作用是对图像进行目标检测,返回一个字符串 info_show。
在函数内部,首先将原始图像 showimg 赋值给变量 showimg。然后使用 letterbox 函数调整图像大小到指定大小(self.opt.img_size),并进行一些预处理操作,将其转换为 PyTorch 的张量格式。接着使用模型 self.model 对图像进行预测,得到预测结果 pred。
对于每个预测结果 det,将其框的坐标进行还原,然后使用 plot_one_box2 函数在图像上绘制出矩形框和标签,并将标签信息添加到 name_list 中。最终将所有标签信息合并为一个字符串 info_show 并返回。
def detect(self, name_list, img): showimg = img with torch.no_grad(): img = letterbox(img, new_shape=self.opt.img_size)[0] img = img[:, :, ::-1].transpose(2, 0, 1) # BGR to RGB, to 3x416x416 img = np.ascontiguousarray(img) img = torch.from_numpy(img).to(self.device) img = img.half() if self.half else img.float() # uint8 to fp16/32 img /= 255.0 # 0 - 255 to 0.0 - 1.0 if img.ndimension() == 3: img = img.unsqueeze(0) pred = self.model(img, augment=self.opt.augment)[0] pred = non_max_suppression(pred, self.opt.conf_thres, self.opt.iou_thres, classes=self.opt.classes, agnostic=self.opt.agnostic_nms) info_show = "" for i, det in enumerate(pred): if det is not None and len(det): det[:, :4] = scale_coords(img.shape[2:], det[:, :4], showimg.shape).round() for *xyxy, conf, cls in reversed(det): label = '%s %.2f' % (self.names[int(cls)], conf) name_list.append(self.names[int(cls)]) single_info = plot_one_box2(xyxy, showimg, label=label, color=self.colors[int(cls)], line_thickness=2) # print(single_info) info_show = info_show + single_info + "\n" return info_show解释代码
这个代码是对输入的图片进行目标检测的,其中包含以下步骤:
1. 将输入的图片进行缩放,使其宽度和高度都等于opt.img_size(一般情况下为416),并将其转换为RGB格式。
2. 将缩放后的图片转换为PyTorch的tensor,并将其从uint8类型转换为fp16或fp32类型,同时将像素值从0-255的范围转换为0.0-1.0的范围。
3. 将tensor的维度变为4维,即增加一个batch维度。
4. 通过调用self.model对图片进行预测,得到预测结果pred。
5. 对pred进行非极大值抑制,去除重复的预测框。
6. 遍历每个预测框,将其坐标进行缩放,使其适应原始图像的大小,并将其类别和置信度添加到name_list中。同时,将每个预测框的坐标和类别信息绘制在原始图像上,并将绘制的信息添加到info_show中。
7. 将info_show返回作为函数的输出结果。
相关推荐
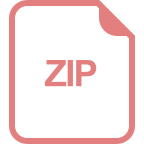














