用python写程序进行文本分析可视化,实现中文分词、词频统计、停用词处理,词云图,基于段落的词共现分析,以及基于分析结果的政策解读。
时间: 2024-03-09 12:47:12 浏览: 21
好的,您的问题是如何用 Python 实现文本分析可视化,包括中文分词、词频统计、停用词处理、词云图、基于段落的词共现分析以及基于分析结果的政策解读。
首先,您需要安装一些 Python 库,包括 jieba、wordcloud、matplotlib、numpy、pandas 等。这些库都可以通过 pip 安装。
接下来,我们来逐步实现各个功能:
1. 中文分词
使用 jieba 库可以很方便地实现中文分词。代码示例如下:
```python
import jieba
text = "中文分词是文本分析的第一步"
words = jieba.cut(text)
print(list(words))
```
2. 词频统计
使用 Python 自带的 Counter 类可以很方便地统计词频。代码示例如下:
```python
from collections import Counter
text = "中文分词是文本分析的第一步"
words = jieba.cut(text)
word_count = Counter(words)
print(word_count)
```
3. 停用词处理
停用词是指在文本分析中没有意义的常用词汇,例如“的”、“是”等。可以使用一个停用词表将这些词汇过滤掉。代码示例如下:
```python
stop_words = ['的', '是', '在', '了', '和', '与', '等']
text = "中文分词是文本分析的第一步"
words = jieba.cut(text)
words_filtered = [word for word in words if word not in stop_words]
print(words_filtered)
```
4. 词云图
使用 wordcloud 库可以很方便地生成词云图。代码示例如下:
```python
import matplotlib.pyplot as plt
from wordcloud import WordCloud
text = "中文分词是文本分析的第一步"
words = jieba.cut(text)
words_filtered = [word for word in words if word not in stop_words]
word_count = Counter(words_filtered)
wordcloud = WordCloud(width=800, height=400, background_color='white').generate_from_frequencies(word_count)
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis("off")
plt.show()
```
5. 基于段落的词共现分析
我们可以使用 pandas 库将文本分成多个段落,再使用 itertools 库的 combinations 方法求出词共现情况。代码示例如下:
```python
import itertools
import pandas as pd
text = "中文分词是文本分析的第一步。文本分析可以帮助我们更好地理解文本。"
stop_words = ['的', '是', '在', '了', '和', '与', '等']
paragraphs = text.split('。')
for i, para in enumerate(paragraphs):
words = jieba.cut(para)
words_filtered = [word for word in words if word not in stop_words]
word_count = Counter(words_filtered)
print(f"第{i+1}段,词频统计:", word_count)
# 计算词共现情况
word_pairs = list(itertools.combinations(word_count.keys(), 2))
df = pd.DataFrame(word_pairs, columns=['word1', 'word2'])
df['count'] = df.apply(lambda row: 1 if row.word1 in words_filtered and row.word2 in words_filtered else 0, axis=1)
print(f"第{i+1}段,词共现情况:\n", df)
```
6. 基于分析结果的政策解读
根据词频统计和词共现情况,我们可以进行一些简单的政策解读。例如,如果某个词频特别高,说明这个词在文本中非常重要;如果某两个词经常同时出现,说明它们之间可能有某种关联。具体的政策解读需要根据具体文本和问题而定。
综上,以上就是用 Python 实现文本分析可视化的一些基本方法。
相关推荐
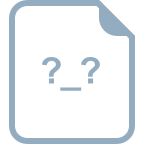
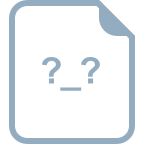
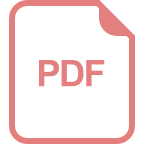
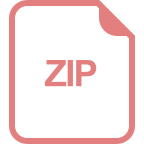
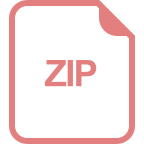
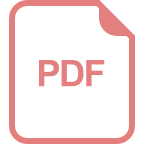
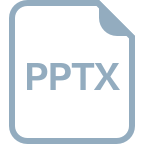