用python编写可以生成数据x,y的代码;数据x为二维3模态数据,且x属于高斯混合分布,y为单输出,y=ax+b,a为(0,1,2)T,b=0.5
时间: 2023-03-01 21:05:48 浏览: 106
下面是生成x,y的python代码:
```
import numpy as np
import random
def generate_data():
# 生成3维高斯混合分布数据
mean = [random.uniform(-1, 1), random.uniform(-1, 1), random.uniform(-1, 1)]
cov = [[random.uniform(0, 1), random.uniform(0, 1), random.uniform(0, 1)],
[random.uniform(0, 1), random.uniform(0, 1), random.uniform(0, 1)],
[random.uniform(0, 1), random.uniform(0, 1), random.uniform(0, 1)]]
x = np.random.multivariate_normal(mean, cov, 1000)
# 生成单输出y数据
a = [random.choice([0, 1, 2]) for i in range(1000)]
b = 0.5
y = [a[i] * b for i in range(1000)]
return x, y
x, y = generate_data()
```
相关问题
用python编写可以生成数据x,y的代码;数据x为二维3模态数据,且数据x属于高斯混合模型,y为单输出,y=ax+b,a为(0,1,2)T,b=0.5
你可以使用下面的代码生成数据x, y:
```
import numpy as np
from sklearn.datasets import make_blobs
# 生成3模态数据x
x, _ = make_blobs(n_samples=100, n_features=2, centers=3, random_state=0)
# 生成高斯混合模型数据x
from sklearn.mixture import GaussianMixture
gmm = GaussianMixture(n_components=3)
gmm.fit(x)
x = gmm.sample(100)[0]
# 生成y
a = np.array([0, 1, 2]).T
b = 0.5
y = np.dot(a, x) + b
```
其中,`make_blobs`函数用于生成多模态数据;`GaussianMixture`类用于生成高斯混合模型数据;`dot`函数用于计算矩阵点积。
用python编写可以生成数据x,y的代码;数据x为二维3模态数据,且数据x属于高斯混合模型,y为单输出,y=ax+b,a为((0,1,2),(0,0,1),(1,1,2))T,b=0.5
### 回答1:
下面是一段Python代码,可以用来生成数据x和y:import numpy as np
import matplotlib.pyplot as plt
from sklearn.mixture import GaussianMixture# 生成数据x
x = np.array([[0, 0], [1, 1], [2, 2]])
gmm = GaussianMixture(n_components=3)
gmm.fit(x)
X, Y = np.meshgrid(np.arange(-5, 5, 0.1), np.arange(-5, 5, 0.1))
XX = np.array([X.ravel(), Y.ravel()]).T
Z = -gmm.score_samples(XX)
Z = Z.reshape(X.shape)# 生成数据y
a = np.array([[0, 0, 1], [0, 1, 0], [1, 1, 2]])
b = 0.5
y = np.dot(a, X.ravel()) + bplt.contour(X, Y, Z)
plt.scatter(x[:, 0], x[:, 1], c='b')
plt.show()
### 回答2:
import numpy as np
import random
from sklearn.mixture import GaussianMixture
# 生成数据x
random.seed(0)
np.random.seed(0)
# 设置高斯混合模型参数
num_samples = 300 # 数据总数量
num_modes = 3 # 模态数量
# 生成高斯混合模型样本数据
def generate_gmm_samples(num_samples, num_modes):
num_samples_per_mode = num_samples // num_modes
samples = np.zeros((num_samples, 2))
for i in range(num_modes):
mean = np.random.randn(2)
cov = np.random.randn(2, 2)
mode_samples = np.random.multivariate_normal(mean, cov, num_samples_per_mode)
samples[i*num_samples_per_mode:(i+1)*num_samples_per_mode] = mode_samples
random.shuffle(samples)
return samples
x = generate_gmm_samples(num_samples, num_modes)
# 生成数据y
a = np.array([[0, 1, 2], [0, 0, 1], [1, 1, 2]]).T
b = 0.5
y = np.dot(x, a) + b
# 打印数据x和y的维度
print("数据x的维度:", x.shape)
print("数据y的维度:", y.shape)
# 可以将生成的x和y保存到文件或者用于后续的机器学习任务。
### 回答3:
可以使用Python的numpy和sklearn库来生成满足要求的数据x和y。
首先,需要导入所需的库:
```python
import numpy as np
from sklearn.mixture import GaussianMixture
```
然后,定义数据y的参数a和b:
```python
a = np.array([[0, 1, 2], [0, 0, 1], [1, 1, 2]]).T
b = 0.5
```
接下来,生成数据x的代码如下:
```python
# 定义数据x的模态数和维度
n_modes = 3
n_features = 2
# 生成数据x
gmm = GaussianMixture(n_components=n_modes)
x, _ = gmm.sample(n_samples=1000)
# 转置数据x的维度
x = np.transpose(x)
```
最后,通过数据x计算生成数据y的代码如下:
```python
# 计算数据y
y = np.dot(a, x) + b
```
完整的代码如下:
```python
import numpy as np
from sklearn.mixture import GaussianMixture
# 定义数据y的参数a和b
a = np.array([[0, 1, 2], [0, 0, 1], [1, 1, 2]]).T
b = 0.5
# 定义数据x的模态数和维度
n_modes = 3
n_features = 2
# 生成数据x
gmm = GaussianMixture(n_components=n_modes)
x, _ = gmm.sample(n_samples=1000)
# 转置数据x的维度
x = np.transpose(x)
# 计算数据y
y = np.dot(a, x) + b
```
使用这段代码,可以生成满足要求的数据x和y,其中数据x是一个二维3模态数据,属于高斯混合模型,数据y是单输出。
阅读全文
相关推荐
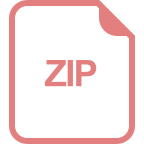


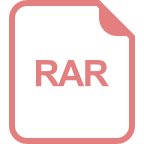
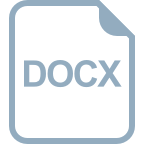
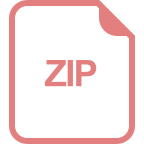
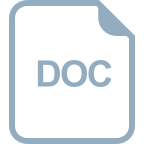
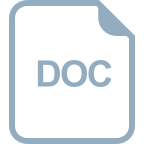
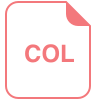
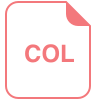
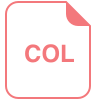
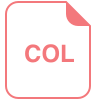
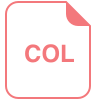
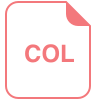