tensorflow显示模型具体结构,并保存成一张图片,写出具体代码
时间: 2023-09-13 22:05:20 浏览: 135
### 回答1:
您可以使用 TensorFlow 的 Keras API 中的 `model.summary()` 方法来查看模型的结构。如果要将模型结构保存为图片,可以使用 `plot_model` 函数。
具体代码如下:
```
from tensorflow import keras
from tensorflow.keras.utils import plot_model
# 定义模型
model = keras.Sequential()
model.add(keras.layers.Dense(32, input_shape=(784,)))
model.add(keras.layers.Dense(10))
# 打印模型结构
model.summary()
# 保存模型结构图片
plot_model(model, to_file='model.png', show_shapes=True)
```
这将会在当前目录下生成一个名为 "model.png" 的图片, 展示模型结构.
### 回答2:
要显示tensorflow模型的具体结构并保存成一张图片,我们可以使用TensorBoard工具。下面是实现这个功能的具体代码:
```python
import tensorflow as tf
from tensorflow.python.platform import gfile
import tensorflow.compat.v1.graph_util as graph_util
from tensorflow.python.framework import graph_io
from tensorflow.summary import FileWriter
# 加载模型
with tf.Session() as sess:
model_filename = 'path_to_your_model/model.pb' # 模型文件路径
with gfile.FastGFile(model_filename, 'rb') as f:
graph_def = tf.GraphDef()
graph_def.ParseFromString(f.read())
tf.import_graph_def(graph_def, name='')
# 保存模型结构图片
graph = tf.get_default_graph()
graph_def = graph.as_graph_def()
summary_writer = FileWriter('path_to_save_image', sess.graph) # 图片保存的路径
summary_writer.add_graph(graph_def)
summary_writer.flush()
print("模型结构已保存为图片")
```
上述代码中,需要将`path_to_your_model/model.pb`替换为你的模型文件路径,`path_to_save_image`替换为保存图片的路径。执行此代码后,模型的结构将被保存为一张图片。
### 回答3:
要显示TensorFlow模型的具体结构,并保存为一张图片,可以使用TensorBoard工具。TensorBoard是TensorFlow提供的一个可视化工具,用于分析和理解模型的结构和计算图。
下面是使用TensorBoard来显示并保存模型结构的代码示例:
```python
import tensorflow as tf
from tensorflow.python.keras.utils import plot_model
# 构建模型
inputs = tf.keras.Input(shape=(784,))
x = tf.keras.layers.Dense(64, activation='relu')(inputs)
x = tf.keras.layers.Dense(64, activation='relu')(x)
outputs = tf.keras.layers.Dense(10, activation='softmax')(x)
model = tf.keras.Model(inputs=inputs, outputs=outputs)
# 保存模型结构为一张图片
plot_model(model, to_file='model.png', show_shapes=True)
```
在代码中,我们首先使用Keras的API建立一个简单的模型,然后使用`plot_model()`函数将模型的结构保存为一张名为`model.png`的图片。`show_shapes=True`参数会显示出每层的输入和输出大小。
运行以上代码后,会在当前目录下生成一张`model.png`图片,图片中展示了模型的结构,包括输入层、中间层和输出层的连接关系。
要使用TensorBoard来可视化模型,可以将生成的图片放入TensorBoard的日志目录中,并在代码中加入如下代码来启动TensorBoard:
```python
tensorboard_callback = tf.keras.callbacks.TensorBoard(log_dir='./logs')
model.fit(x_train, y_train, epochs=10, validation_data=(x_test, y_test), callbacks=[tensorboard_callback])
```
然后在命令行中运行`tensorboard --logdir=./logs`,然后在浏览器中打开生成的链接,即可在TensorBoard中查看模型的结构和其他统计信息。
相关推荐
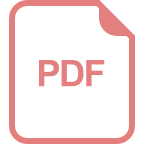
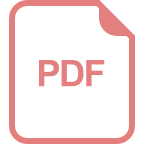














