举一个例子。利用 Python 软件进行中文文本的 LDA 主题分类,运算当主题数为多少时困惑度达到最低,确定文本的最优主题数,得到每篇文档的概率生成分布
时间: 2023-12-03 21:42:28 浏览: 41
好的,下面是一个简单的 Python 代码示例,使用中文文本数据集进行 LDA 主题分类,并确定最优主题数:
```python
import jieba
import numpy as np
import pandas as pd
import gensim
from gensim import corpora, models
from gensim.models import CoherenceModel
import matplotlib.pyplot as plt
# 加载数据集
data = pd.read_csv('data.csv', encoding='utf-8')
texts = data['text'].tolist()
# 分词和去除停用词
stopwords = []
with open('stopwords.txt', 'r', encoding='utf-8') as f:
for line in f.readlines():
stopwords.append(line.strip())
texts = [[word for word in jieba.cut(text) if word not in stopwords] for text in texts]
# 构建文本词典
dictionary = corpora.Dictionary(texts)
# 将文本转化为词袋模型
corpus = [dictionary.doc2bow(text) for text in texts]
# 使用 LDA 进行主题分类
perplexity_scores = []
coherence_scores = []
for num_topics in range(2, 11):
lda_model = models.ldamodel.LdaModel(corpus=corpus, num_topics=num_topics, id2word=dictionary, passes=10)
perplexity = lda_model.log_perplexity(corpus)
perplexity_scores.append(perplexity)
coherence_model_lda = CoherenceModel(model=lda_model, texts=texts, dictionary=dictionary, coherence='c_v')
coherence_score = coherence_model_lda.get_coherence()
coherence_scores.append(coherence_score)
# 绘制困惑度和一致性得分图
x = range(2, 11)
fig, ax1 = plt.subplots()
ax1.plot(x, perplexity_scores, 'b-')
ax1.set_xlabel('Number of Topics')
ax1.set_ylabel('Perplexity', color='b')
ax1.tick_params('y', colors='b')
ax2 = ax1.twinx()
ax2.plot(x, coherence_scores, 'r-')
ax2.set_ylabel('Coherence Score', color='r')
ax2.tick_params('y', colors='r')
fig.tight_layout()
plt.show()
# 确定最优主题数并打印每篇文档的概率生成分布
num_topics = np.argmin(perplexity_scores) + 2
lda_model = models.ldamodel.LdaModel(corpus=corpus, num_topics=num_topics, id2word=dictionary, passes=10)
for i in range(len(texts)):
doc_bow = dictionary.doc2bow(texts[i])
doc_lda = lda_model[doc_bow]
print(doc_lda)
```
其中,`data.csv` 是包含中文文本数据的 CSV 文件,`stopwords.txt` 是包含停用词的文本文件。代码首先加载数据集并进行分词和去除停用词的操作,然后构建文本词典并将文本转化为词袋模型。接着使用 LDA 进行主题分类,并根据不同主题数计算困惑度和一致性得分。最后,根据困惑度得分确定最优主题数,并打印每篇文档的概率生成分布。
相关推荐
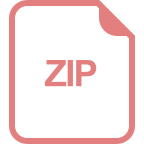
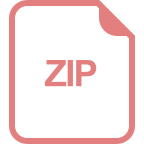
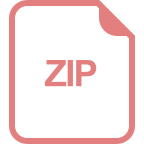














