python计算图像信息熵
时间: 2023-06-02 18:03:19 浏览: 229
好的,关于计算图像信息熵的问题,我可以尝试回答。在使用Python处理图像时,可以使用PIL库或OpenCV库来读取和处理图像。计算图像信息熵时,可以使用scipy库的entropy函数来实现。具体实现方法是先将图像转换为灰度图像,然后通过entropy函数计算信息熵。
相关问题
python计算彩色图像信息熵
以下是使用Python计算彩色图像信息熵的示例代码:
```python
import numpy as np
from PIL import Image
# 读取图像
img = Image.open('image.png')
# 转换为numpy数组
img_arr = np.array(img)
# 计算每个像素点的信息熵
h, w, c = img_arr.shape
entropy = np.zeros((h, w))
for i in range(h):
for j in range(w):
# 计算每个通道的直方图
hist_r, _ = np.histogram(img_arr[i,j,0], bins=256, range=(0,255))
hist_g, _ = np.histogram(img_arr[i,j,1], bins=256, range=(0,255))
hist_b, _ = np.histogram(img_arr[i,j,2], bins=256, range=(0,255))
# 计算每个通道的概率分布
p_r = hist_r / np.sum(hist_r)
p_g = hist_g / np.sum(hist_g)
p_b = hist_b / np.sum(hist_b)
# 计算信息熵
entropy[i,j] = -np.sum(p_r*np.log2(p_r+1e-6)) \
-np.sum(p_g*np.log2(p_g+1e-6)) \
-np.sum(p_b*np.log2(p_b+1e-6))
# 计算平均信息熵
mean_entropy = np.mean(entropy)
print('平均信息熵:', mean_entropy)
```
需要注意的是,在计算信息熵时,需要对概率分布中可能出现的0值进行平滑,以避免计算结果出现NaN。在上面的代码中,使用了一个很小的常数1e-6进行平滑。
从python opencv中计算信息熵
要从Python OpenCV中计算信息熵,您需要首先将图像转换为灰度图像,然后使用NumPy计算像素值的频率分布。接下来,您可以使用SciPy中的熵函数来计算信息熵。
下面是一个简单的示例代码:
```python
import cv2
import numpy as np
from scipy.stats import entropy
# 读取图像并转换为灰度图像
img = cv2.imread('image.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 计算像素值的频率分布
hist, _ = np.histogram(gray.ravel(), bins=256, range=[0,256])
hist = hist / float(np.sum(hist))
# 计算图像的信息熵
entropy = entropy(hist, base=2)
print('图像信息熵为:', entropy)
```
在这里,我们首先读取图像并将其转换为灰度图像。然后,我们使用NumPy的histogram函数计算像素值的频率分布。最后,我们使用SciPy的熵函数计算图像的信息熵,并将其打印出来。
请注意,我们在计算熵时使用了2作为基数,这意味着我们使用比特作为单位来度量信息量。如果您希望使用其他基数,可以将“base”参数设置为所需的值。
相关推荐
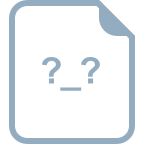
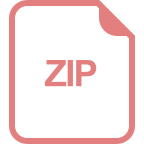












