C语言指定目录有两个文本文件,父和子分别对两个文件进行统计 父进程统计文件a中数字的个数 子进程统计文件b中字母的个数
时间: 2023-06-04 11:02:49 浏览: 45
父进程可以使用C语言中的文件操作函数fopen()打开文件a,然后使用getc()函数逐个读取文件中的字符,使用isdigit()函数判断字符是否为数字,统计数字的个数即可。
子进程可以同样使用fopen()函数打开文件b,使用getc()函数逐个读取文件中的字符,使用isalpha()函数判断字符是否为字母,统计字母的个数即可。
代码示例:
```
#include <stdio.h>
#include <ctype.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
int count_digits(FILE *file) {
int count = 0;
int c;
while ((c = getc(file)) != EOF) {
if (isdigit(c)) {
count++;
}
}
return count;
}
int count_letters(FILE *file) {
int count = 0;
int c;
while ((c = getc(file)) != EOF) {
if (isalpha(c)) {
count++;
}
}
return count;
}
int main() {
int pid = fork();
if (pid == 0) {
// child process
FILE *file = fopen("b.txt", "r");
int count = count_letters(file);
printf("Number of letters in b.txt: %d\n", count);
fclose(file);
} else {
// parent process
FILE *file = fopen("a.txt", "r");
int count = count_digits(file);
printf("Number of digits in a.txt: %d\n", count);
fclose(file);
wait(NULL); // wait for child process
}
return 0;
}
```
相关推荐
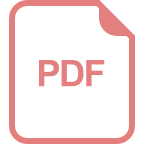
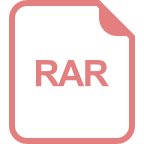














