python字典转字符串
时间: 2023-09-19 16:06:20 浏览: 101
Python 字典可以通过 str() 函数转换为字符串,也可以使用 json 模块中的 dumps() 函数将字典转换为 JSON 格式的字符串。
使用 str() 函数:
```
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
my_str = str(my_dict)
print(my_str)
```
输出:
```
{'name': 'Alice', 'age': 25, 'city': 'New York'}
```
使用 json.dumps() 函数:
```
import json
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
my_str = json.dumps(my_dict)
print(my_str)
```
输出:
```
{"name": "Alice", "age": 25, "city": "New York"}
```
相关问题
python字典统计字符串出现次数
可以使用python的字典来统计字符串中每个字符出现的次数。
例如,假设有一个字符串s:
```python
s = "hello world"
```
我们可以使用一个字典来统计每个字符出现的次数:
```python
count = {}
for char in s:
if char in count:
count[char] += 1
else:
count[char] = 1
```
最终得到的字典count将包含每个字符出现的次数:
```python
{'h': 1, 'e': 1, 'l': 3, 'o': 2, ' ': 1, 'w': 1, 'r': 1, 'd': 1}
```
我们可以使用dict.items()方法来遍历字典,并输出每个字符出现的次数:
```python
for char, count in count.items():
print(char, count)
```
输出为:
```
h 1
e 1
l 3
o 2
1
w 1
r 1
d 1
```
用PYTHON写一个字典工具类,包括以下字典格式转JSON格式,JSON转字典格式,字典转字符串格式,字符转字典格式
### 回答1:
可以使用 Python 的内置 json 模块来实现这些功能。
这是一个例子:
```
import json
class DictionaryUtils:
@staticmethod
def dict_to_json(dictionary: dict) -> str:
"""将字典转换为 JSON 格式的字符串"""
return json.dumps(dictionary)
@staticmethod
def json_to_dict(json_str: str) -> dict:
"""将 JSON 格式的字符串转换为字典"""
return json.loads(json_str)
@staticmethod
def dict_to_string(dictionary: dict) -> str:
"""将字典转换为字符串格式"""
return str(dictionary)
@staticmethod
def string_to_dict(string: str) -> dict:
"""将字符串转换为字典格式"""
return eval(string)
```
使用方法:
```
# 创建字典
dictionary = {'name': 'Alice', 'age': 20}
# 将字典转换为 JSON 格式的字符串
json_str = DictionaryUtils.dict_to_json(dictionary)
print(json_str) # {"name": "Alice", "age": 20}
# 将 JSON 格式的字符串转换为字典
dictionary = DictionaryUtils.json_to_dict(json_str)
print(dictionary) # {'name': 'Alice', 'age': 20}
# 将字典转换为字符串格式
string = DictionaryUtils.dict_to_string(dictionary)
print(string) # {'name': 'Alice', 'age': 20}
# 将字符串转换为字典格式
dictionary = DictionaryUtils.string_to_dict(string)
print(dictionary) # {'name': 'Alice', 'age': 20}
```
### 回答2:
可以使用Python的内置库`json`来实现字典和JSON格式的相互转换。
首先是将字典格式转换为JSON格式的方法:
```python
import json
def dict_to_json(dictionary):
json_str = json.dumps(dictionary)
return json_str
```
接下来是将JSON格式转换为字典格式的方法:
```python
import json
def json_to_dict(json_str):
dictionary = json.loads(json_str)
return dictionary
```
然后是将字典转换为字符串格式的方法:
```python
def dict_to_str(dictionary):
str_dict = str(dictionary)
return str_dict
```
最后是将字符串格式转换为字典格式的方法:
```python
import ast
def str_to_dict(str_dict):
dictionary = ast.literal_eval(str_dict)
return dictionary
```
以上就是一个使用Python编写的字典工具类,包括了字典格式转JSON格式、JSON转字典格式、字典转字符串格式和字符串转字典格式的功能。基于以上代码,你可以编写一个包含这些方法的工具类,并在需要的时候调用相应的方法。
### 回答3:
可以使用python的json库来完成字典和JSON之间的转换,以及字典和字符串之间的转换。下面是一个简单的字典工具类示例:
```python
import json
class DictUtils:
@staticmethod
def dict_to_json(dictionary):
return json.dumps(dictionary)
@staticmethod
def json_to_dict(json_string):
return json.loads(json_string)
@staticmethod
def dict_to_string(dictionary):
return str(dictionary)
@staticmethod
def string_to_dict(string):
return eval(string)
```
其中,`dict_to_json`方法用于将字典转换为JSON格式,`json_to_dict`方法用于将JSON字符串转换为字典格式,`dict_to_string`方法用于将字典转换为字符串格式,`string_to_dict`方法用于将字符串转换为字典格式。
示例用法如下:
```python
data = {
"name": "John",
"age": 30,
"city": "New York"
}
json_data = DictUtils.dict_to_json(data)
print(json_data) # 输出: {"name": "John", "age": 30, "city": "New York"}
dict_data = DictUtils.json_to_dict(json_data)
print(dict_data) # 输出: {'name': 'John', 'age': 30, 'city': 'New York'}
string_data = DictUtils.dict_to_string(data)
print(string_data) # 输出: {'name': 'John', 'age': 30, 'city': 'New York'}
dict_data = DictUtils.string_to_dict(string_data)
print(dict_data) # 输出: {'name': 'John', 'age': 30, 'city': 'New York'}
```
注意:对于`string_to_dict`方法,在将字符串转换为字典时,使用了`eval`函数,这可能存在一定的安全风险。如果需要更加安全的转换方法,建议使用其他的字典转换工具类。
阅读全文
相关推荐
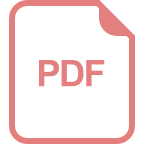
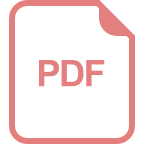
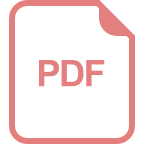
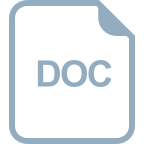
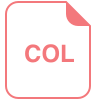







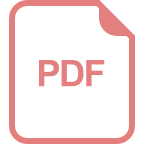
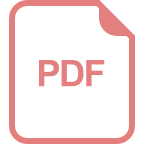