帮我写一段基于棋盘格的位姿提取算法的python代码
时间: 2023-12-29 18:03:51 浏览: 22
好的,以下是一个简单的基于棋盘格的位姿提取算法的Python代码:
```
import cv2
import numpy as np
# 生成棋盘格
width = 8
height = 6
square_size = 1.0 # 棋盘格每个小方格的大小
obj_points = np.zeros((width*height, 3), np.float32)
obj_points[:, :2] = np.mgrid[0:width, 0:height].T.reshape(-1, 2) * square_size
# 读取图像
img = cv2.imread('chessboard.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 查找角点
ret, corners = cv2.findChessboardCorners(gray, (width, height), None)
if ret:
# 绘制角点
cv2.drawChessboardCorners(img, (width, height), corners, ret)
# 计算位姿
ret, rvecs, tvecs = cv2.solvePnP(obj_points, corners, camera_matrix, dist_coeff)
# 输出位姿
print("rvecs:", rvecs)
print("tvecs:", tvecs)
# 绘制坐标系
img_points, _ = cv2.projectPoints(np.array([(0,0,0),(0,0,-1),(0,1,0),(1,0,0)],np.float32), rvecs, tvecs, camera_matrix, dist_coeff)
p1 = tuple(map(int, corners[0].ravel()))
p2 = tuple(map(int, img_points[1].ravel()))
p3 = tuple(map(int, img_points[2].ravel()))
p4 = tuple(map(int, img_points[3].ravel()))
cv2.line(img, p1, p2, (0, 0, 255), 2)
cv2.line(img, p1, p3, (0, 255, 0), 2)
cv2.line(img, p1, p4, (255, 0, 0), 2)
# 显示结果
cv2.imshow('result', img)
cv2.waitKey(0)
else:
print("Not found chessboard corners!")
```
请注意,这段代码中的 `camera_matrix` 和 `dist_coeff` 需要根据你的相机进行调整。
相关推荐
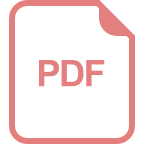














