python re模块有什么方法可以获取到match方法返回对象中的匹配的字符串
时间: 2024-03-27 22:39:24 浏览: 61
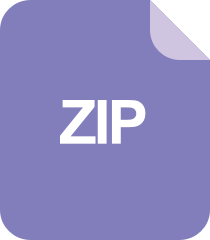
fuzzy_matcher:使用 Levenshtein 距离算法进行近似字符串匹配
可以使用Match对象的group方法来获取匹配的字符串。例如,假设我们有一个正则表达式r'\d+',并且我们使用它来匹配字符串"12345",则可以使用以下代码来获取匹配的字符串:
```
import re
pattern = r'\d+'
text = "12345"
match_obj = re.match(pattern, text)
matched_str = match_obj.group()
print(matched_str) # 输出 "12345"
```
在这个例子中,我们使用re.match方法匹配了字符串"12345",并且得到了一个Match对象match_obj。然后,我们调用了match_obj的group方法来获取匹配的字符串"12345"。
阅读全文
相关推荐
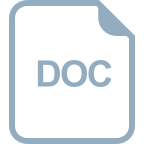
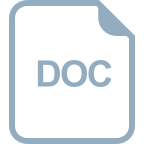
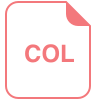
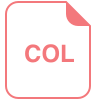
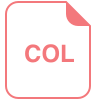










