用C语言模拟实现先来先服务(FCFS)、最短寻道优先(SSTF)、电梯算法(SCAN、方向为从低磁道向高磁道)三种磁盘调度算法的磁道访问过程。
时间: 2024-05-07 20:09:55 浏览: 14
这是一个比较复杂的问题,需要编写一定量的代码才能实现。下面给出一个简单的示例代码,仅供参考。
首先,我们需要定义一个磁道请求结构体,用来存储每个请求的信息:
```c
struct request {
int track; // 磁道号
int visited; // 是否已访问过
};
```
然后,我们需要实现三种磁盘调度算法的函数。这里我们先实现 FCFS 算法:
```c
void fcfs(struct request *req, int n) {
int current = 0; // 当前磁头所在磁道
int total_distance = 0; // 总寻道距离
for (int i = 0; i < n; i++) {
int distance = abs(req[i].track - current); // 计算到达下一个请求的距离
total_distance += distance; // 累加总寻道距离
current = req[i].track; // 更新当前磁头所在磁道
}
printf("FCFS: Total distance = %d\n", total_distance);
}
```
SSTF 算法比较复杂,需要对请求进行排序。这里我们采用冒泡排序,但实际上更高效的排序算法可以提高程序的性能:
```c
void sstf(struct request *req, int n) {
int current = 0; // 当前磁头所在磁道
int total_distance = 0; // 总寻道距离
for (int i = 0; i < n; i++) {
// 选择距离当前磁头最近的请求
int nearest = -1;
int min_distance = INT_MAX;
for (int j = 0; j < n; j++) {
if (!req[j].visited) {
int distance = abs(req[j].track - current);
if (distance < min_distance) {
nearest = j;
min_distance = distance;
}
}
}
// 更新当前磁头位置和总寻道距离
req[nearest].visited = 1;
total_distance += min_distance;
current = req[nearest].track;
}
printf("SSTF: Total distance = %d\n", total_distance);
}
```
最后是 SCAN 算法。这里我们假设磁头方向为从低磁道向高磁道,因此需要将请求按照磁道号从小到大排序:
```c
void scan(struct request *req, int n) {
int current = 0; // 当前磁头所在磁道
int total_distance = 0; // 总寻道距离
int direction = 1; // 磁头移动方向:1 表示向高磁道,-1 表示向低磁道
// 按磁道号排序
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - 1 - i; j++) {
if (req[j].track > req[j+1].track) {
struct request tmp = req[j];
req[j] = req[j+1];
req[j+1] = tmp;
}
}
}
// 找到第一个大于当前磁头位置的请求
int next = 0;
while (next < n && req[next].track < current) {
next++;
}
while (next < n || direction == 1) {
// 计算到达下一个请求的距离
int distance = 0;
if (next < n) {
distance = abs(req[next].track - current);
} else {
distance = abs(199 - current);
}
// 更新当前磁头位置和总寻道距离
total_distance += distance;
current += direction * distance;
// 判断是否到达边界,需要改变方向
if (current == 0 || current == 199) {
direction = -direction;
}
// 找到下一个请求
while (next < n && req[next].track <= current) {
next++;
}
}
printf("SCAN: Total distance = %d\n", total_distance);
}
```
完整代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <limits.h>
struct request {
int track;
int visited;
};
void fcfs(struct request *req, int n) {
int current = 0;
int total_distance = 0;
for (int i = 0; i < n; i++) {
int distance = abs(req[i].track - current);
total_distance += distance;
current = req[i].track;
}
printf("FCFS: Total distance = %d\n", total_distance);
}
void sstf(struct request *req, int n) {
int current = 0;
int total_distance = 0;
for (int i = 0; i < n; i++) {
int nearest = -1;
int min_distance = INT_MAX;
for (int j = 0; j < n; j++) {
if (!req[j].visited) {
int distance = abs(req[j].track - current);
if (distance < min_distance) {
nearest = j;
min_distance = distance;
}
}
}
req[nearest].visited = 1;
total_distance += min_distance;
current = req[nearest].track;
}
printf("SSTF: Total distance = %d\n", total_distance);
}
void scan(struct request *req, int n) {
int current = 0;
int total_distance = 0;
int direction = 1;
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - 1 - i; j++) {
if (req[j].track > req[j+1].track) {
struct request tmp = req[j];
req[j] = req[j+1];
req[j+1] = tmp;
}
}
}
int next = 0;
while (next < n && req[next].track < current) {
next++;
}
while (next < n || direction == 1) {
int distance = 0;
if (next < n) {
distance = abs(req[next].track - current);
} else {
distance = abs(199 - current);
}
total_distance += distance;
current += direction * distance;
if (current == 0 || current == 199) {
direction = -direction;
}
while (next < n && req[next].track <= current) {
next++;
}
}
printf("SCAN: Total distance = %d\n", total_distance);
}
int main() {
struct request req[] = {
{ 55, 0 },
{ 58, 0 },
{ 39, 0 },
{ 18, 0 },
{ 90, 0 },
{ 160, 0 },
{ 150, 0 },
{ 38, 0 },
{ 184, 0 },
{ 65, 0 },
};
int n = sizeof(req) / sizeof(req[0]);
fcfs(req, n);
for (int i = 0; i < n; i++) {
req[i].visited = 0;
}
sstf(req, n);
for (int i = 0; i < n; i++) {
req[i].visited = 0;
}
scan(req, n);
return 0;
}
```
相关推荐
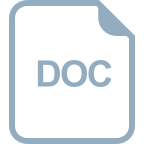
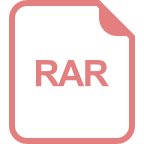








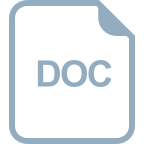
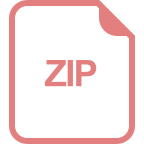
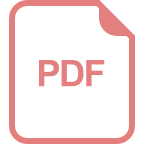
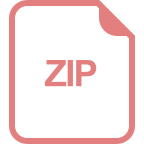