磁盘调度算法编程实现先来先服务算法(FCFS)、最短寻道时间优先算法(SSTF)、扫描算法(SCAN)、循环扫描算法(CSCAN)等。
时间: 2024-07-28 09:00:29 浏览: 82
磁盘调度算法是操作系统中用于优化磁盘I/O操作性能的重要技术,不同的算法基于不同的策略来决定哪个请求应该优先执行。以下是几种常见的磁盘调度算法:
1. 先来先服务(FCFS, First-Come-First-Served): 这是最简单的调度算法,按照请求到达的顺序进行处理,不考虑磁头的位置。每个新请求到达后,都会被添加到队列的末尾,磁头会一直移动到队列的头部开始服务,直到该请求完成。
2. 最短寻道时间优先(SSTF, ShortestSeekTimeFirst): SSTF算法的目标是尽快响应最近的请求。它每次选择当前磁头位置到下一个请求的起始位置路径最短的请求。但这种算法可能导致磁头频繁移动,可能导致其他请求等待时间过长。
3. 扫描算法(SCAN): SCAN算法从一个固定的位置出发,沿着磁道的某一方向进行扫描,直到队列为空或磁头到达磁盘的边缘,然后返回起点继续扫描。这减少了磁头移动的总距离,提高了效率,但也可能造成磁头长时间停在某些区域。
4. 循环扫描算法(CSCAN, Cylindrical SCAN): 类似于SCAN,但不是沿单一方向扫描,而是沿着圆周方向在一个磁道范围内移动,这样可以更均匀地分布I/O请求,避免了单向扫描可能导致的热点问题。
编程实现这些算法时,通常涉及维护一个请求队列,磁头状态,以及根据不同的算法逻辑进行决策和更新。每种算法都需要设计适当的逻辑来选择下一个访问的磁道和扇区,例如在FCFS中,只需按顺序处理;而在SSTF中,可能需要维护一个最小寻道距离的数据结构。
相关问题
磁盘调度算法c语言代码包括先来先服务(FCFS)、最短寻道时间优先(SSTF)、扫描算法(SCAN)
以下FCFS、SSTF和SCAN算法的C语言代码:
1. 先来先服务(FCFS)算法:
```c
#include <stdio.h>
#include <stdlib.h>
int main()
{
int n, i, j, sum = 0, head, req[100];
printf("Enter the number of requests: ");
scanf("%d", &n);
printf("Enter the requests: ");
for(i=0; i<n; i++)
scanf("%d", &req[i]);
printf("Enter the initial head position: ");
scanf("%d", &head);
printf("The order of servicing the requests is:\n%d ", head);
for(i=0; i<n; i++)
{
printf("%d ", req[i]);
sum += abs(head - req[i]);
head = req[i];
}
printf("\nTotal head movements: %d", sum);
return 0;
}
```
2. 最短寻道时间优先(SSTF)算法:
```c
#include <stdio.h>
#include <stdlib.h>
int main()
{
int n, i, j, sum = 0, head, min, diff, pos, req[100], flag[100]={0};
printf("Enter the number of requests: ");
scanf("%d", &n);
printf("Enter the requests: ");
for(i=0; i<n; i++)
scanf("%d", &req[i]);
printf("Enter the initial head position: ");
scanf("%d", &head);
printf("The order of servicing the requests is:\n%d ", head);
for(i=0; i<n; i++)
{
min = 1000;
for(j=0; j<n; j++)
{
if(flag[j]==0)
{
diff = abs(head - req[j]);
if(diff < min)
{
min = diff;
pos = j;
}
}
}
sum += abs(head - req[pos]);
head = req[pos];
flag[pos] = 1;
printf("%d ", req[pos]);
}
printf("\nTotal head movements: %d", sum);
return 0;
}
```
3. 扫描算法(SCAN):
```c
#include <stdio.h>
#include <stdlib.h>
int main()
{
int n, i, j, sum = 0, head, max, k, req[100], flag[100];
printf("Enter the number of requests: ");
scanf("%d", &n);
printf("Enter the requests: ");
for(i=0; i<n; i++)
scanf("%d", &req[i]);
printf("Enter the initial head position: ");
scanf("%d", &head);
printf("Enter the maximum cylinder size: ");
scanf("%d", &max);
printf("The order of servicing the requests is:\n");
for(i=head; i<=max; i++)
flag[i] = 0;
for(i=0; i<n; i++)
flag[req[i]] = 1;
for(i=head; i>=0; i--)
flag[i] = 0;
sum = head;
printf("%d ", head);
for(i=head; i<=max; i++)
{
if(flag[i]==1)
{
printf("%d ", i);
sum += abs(head - i);
head = i;
}
}
for(i=max; i>=0; i--)
{
if(flag[i]==1)
{
printf("%d ", i);
sum += abs(head - i);
head = i;
}
}
printf("\nTotal head movements: %d", sum);
return 0;
}
```
上述代码分别实现了FCFS、SSTF和SCAN三种磁盘调度算法,您可以根据需要选择其中的一种或多种进行使用。
写代码 通过这次实验,加深对磁盘调度算法的理解,进一步掌握先来先服务FCFS,最短寻道时间优先SSTF,SCAN和循环SCAN算法的实现方法。
好,下面我将为你介绍一下这四种磁盘调度算法的实现方法。
## FCFS算法
FCFS(First Come First Serve)算法是最简单的磁盘调度算法,它按照磁盘请求的到达时间顺序进行服务。具体实现方法如下:
```python
def fcfs(requests, start):
total_distance = 0
current = start
for request in requests:
total_distance += abs(request - current)
current = request
return total_distance
```
其中,`requests`是一个列表,表示磁盘的请求序列,`start`表示磁头的初始位置。`current`表示当前磁头的位置,每次服务完一个请求后,磁头就移动到下一个请求的位置,直到服务完所有请求。`abs(request - current)`表示当前请求和磁头的距离。
## SSTF算法
SSTF(Shortest Seek Time First)算法是一种贪心算法,它每次选择距离磁头最近的请求进行服务。具体实现方法如下:
```python
def sstf(requests, start):
total_distance = 0
current = start
while requests:
# 找到距离当前位置最近的请求
closest = min(requests, key=lambda x: abs(x - current))
total_distance += abs(closest - current)
current = closest
requests.remove(closest)
return total_distance
```
其中,`min(requests, key=lambda x: abs(x - current))`表示找到距离当前位置最近的请求。每次服务完一个请求后,需要从请求序列中将该请求删除。
## SCAN算法
SCAN算法是一种往返扫描算法,它先沿一个方向(一般是向外)扫描磁盘上的请求,直到遇到最远的请求,然后改变方向,沿另一个方向扫描请求,直到遇到最远的请求,如此往返扫描,直到服务完所有请求。具体实现方法如下:
```python
def scan(requests, start, direction):
total_distance = 0
current = start
while requests:
# 找到当前方向上最远的请求
farthest = None
for request in requests:
if (direction == 1 and request >= current) or (direction == -1 and request <= current):
if farthest is None or abs(request - current) > abs(farthest - current):
farthest = request
if farthest is None:
# 没有请求了,需要改变方向
direction = -direction
continue
total_distance += abs(farthest - current)
current = farthest
requests.remove(farthest)
return total_distance
```
其中,`direction`表示扫描的方向,1表示向外扫描,-1表示向内扫描。每次服务完一个请求后,需要判断是否需要改变方向,如果当前方向上没有请求了,则需要改变方向。
## 循环SCAN算法
循环SCAN算法是SCAN算法的变种,它在磁盘的两个端点之间来回扫描,直到服务完所有请求。具体实现方法如下:
```python
def cscan(requests, start):
total_distance = 0
current = start
while requests:
# 找到当前方向上最远的请求
farthest = None
for request in requests:
if request >= current:
if farthest is None or abs(request - current) > abs(farthest - current):
farthest = request
if farthest is None:
# 没有请求了,需要移到磁盘起点
total_distance += abs(199 - current)
current = 0
continue
total_distance += abs(farthest - current)
current = farthest
requests.remove(farthest)
return total_distance
```
其中,如果当前方向上没有请求了,则需要将磁头移到磁盘的起点,重新开始扫描。
以上就是四种磁盘调度算法的实现方法,你可以在实际应用中根据需要选择适合的算法。
阅读全文
相关推荐
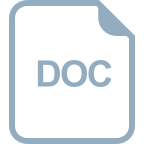
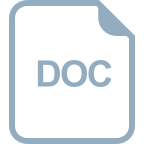
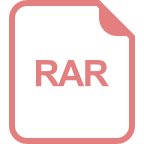
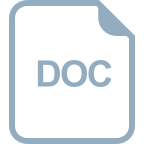












