先来先服务FCFS、最短寻道时间优先SSTF、SCAN、CSCAN算法。
时间: 2023-09-16 16:14:47 浏览: 112
这是一个关于磁盘调度算法的问题。磁盘调度算法是为了优化磁盘读写操作的效率而设计的。常见的磁盘调度算法有先来先服务(FCFS)、最短寻道时间优先(SSTF)、SCAN和CSCAN。
1. 先来先服务(FCFS):按照磁盘请求的顺序进行调度,先发出请求的先被服务,不考虑磁头移动的距离和时间。
2. 最短寻道时间优先(SSTF):选择距离当前磁头最近的请求进行服务,减少磁头的移动时间,提高磁盘的读写效率。
3. SCAN:磁头按照一个方向移动,直到到达磁盘的一端,然后改变方向继续移动,直到服务完所有等待的请求。
4. CSCAN:类似于SCAN,磁头也按照一个方向移动,直到到达磁盘的一端,但是它不会改变方向,而是返回到磁盘的另一端,继续移动,直到服务完所有等待的请求。
这些算法的选择取决于磁盘的使用场景和性能要求。
相关问题
写代码 通过这次实验,加深对磁盘调度算法的理解,进一步掌握先来先服务FCFS,最短寻道时间优先SSTF,SCAN和循环SCAN算法的实现方法。
好,下面我将为你介绍一下这四种磁盘调度算法的实现方法。
## FCFS算法
FCFS(First Come First Serve)算法是最简单的磁盘调度算法,它按照磁盘请求的到达时间顺序进行服务。具体实现方法如下:
```python
def fcfs(requests, start):
total_distance = 0
current = start
for request in requests:
total_distance += abs(request - current)
current = request
return total_distance
```
其中,`requests`是一个列表,表示磁盘的请求序列,`start`表示磁头的初始位置。`current`表示当前磁头的位置,每次服务完一个请求后,磁头就移动到下一个请求的位置,直到服务完所有请求。`abs(request - current)`表示当前请求和磁头的距离。
## SSTF算法
SSTF(Shortest Seek Time First)算法是一种贪心算法,它每次选择距离磁头最近的请求进行服务。具体实现方法如下:
```python
def sstf(requests, start):
total_distance = 0
current = start
while requests:
# 找到距离当前位置最近的请求
closest = min(requests, key=lambda x: abs(x - current))
total_distance += abs(closest - current)
current = closest
requests.remove(closest)
return total_distance
```
其中,`min(requests, key=lambda x: abs(x - current))`表示找到距离当前位置最近的请求。每次服务完一个请求后,需要从请求序列中将该请求删除。
## SCAN算法
SCAN算法是一种往返扫描算法,它先沿一个方向(一般是向外)扫描磁盘上的请求,直到遇到最远的请求,然后改变方向,沿另一个方向扫描请求,直到遇到最远的请求,如此往返扫描,直到服务完所有请求。具体实现方法如下:
```python
def scan(requests, start, direction):
total_distance = 0
current = start
while requests:
# 找到当前方向上最远的请求
farthest = None
for request in requests:
if (direction == 1 and request >= current) or (direction == -1 and request <= current):
if farthest is None or abs(request - current) > abs(farthest - current):
farthest = request
if farthest is None:
# 没有请求了,需要改变方向
direction = -direction
continue
total_distance += abs(farthest - current)
current = farthest
requests.remove(farthest)
return total_distance
```
其中,`direction`表示扫描的方向,1表示向外扫描,-1表示向内扫描。每次服务完一个请求后,需要判断是否需要改变方向,如果当前方向上没有请求了,则需要改变方向。
## 循环SCAN算法
循环SCAN算法是SCAN算法的变种,它在磁盘的两个端点之间来回扫描,直到服务完所有请求。具体实现方法如下:
```python
def cscan(requests, start):
total_distance = 0
current = start
while requests:
# 找到当前方向上最远的请求
farthest = None
for request in requests:
if request >= current:
if farthest is None or abs(request - current) > abs(farthest - current):
farthest = request
if farthest is None:
# 没有请求了,需要移到磁盘起点
total_distance += abs(199 - current)
current = 0
continue
total_distance += abs(farthest - current)
current = farthest
requests.remove(farthest)
return total_distance
```
其中,如果当前方向上没有请求了,则需要将磁头移到磁盘的起点,重新开始扫描。
以上就是四种磁盘调度算法的实现方法,你可以在实际应用中根据需要选择适合的算法。
设计程序模拟先来先服务FCFS,最短寻道时间优先SSTF,SCAN和循环SCAN算法的工作过程。假设有n个磁道号所组成的磁道访问序列,给定开始磁道号m和磁头移动的方向(正向或者反向),分别利用不同的磁盘调度算法访问磁道序列,给出每一次访问的磁头移动距离,计算每种算法的平均寻道长度。
好的,这是一个关于磁盘调度算法的问题,我会尽力解答。下面是对于题目的解析和实现思路:
1. 算法介绍
先来先服务(FCFS)算法:按照磁盘请求的顺序进行调度,先请求的先服务,不考虑磁头移动距离。
最短寻道时间优先(SSTF)算法:选择离当前磁头位置最近的请求进行服务,每次选择完请求后都要更新磁头位置。
SCAN算法:也称为电梯扫描算法,磁头按照一个方向移动,直到到达最边缘,然后改变方向移动,直到服务完所有的请求。
循环SCAN算法:类似SCAN算法,但是不是到达最边缘就改变方向,而是到达最边缘后返回到另一端,再继续服务请求。
2. 实现思路
首先,根据题目给定的请求序列和磁头位置,按照不同的算法排序。然后,根据排序后的请求序列,计算每次移动的距离,最后求出平均寻道长度。
具体实现可以采用以下步骤:
1) 根据题目给定的请求序列和磁头位置,将请求序列按照FCFS、SSTF、SCAN、循环SCAN算法排序。
2) 计算每次移动的距离,记录下来。
3) 计算平均寻道长度,即所有移动距离之和除以请求总数。
下面是伪代码实现:
```
// 输入参数
// n:磁道请求序列长度
// m:磁头位置
// direction:磁头移动方向,1表示正向,-1表示反向
// seq[]:磁道请求序列
// FCFS算法
fcfs(seq, n, m, direction) {
int move = 0; // 记录移动距离
int curr = m; // 记录当前磁头位置
for (int i = 0; i < n; i++) {
move += abs(curr - seq[i]); // 计算移动距离
curr = seq[i]; // 更新磁头位置
}
return move / n; // 返回平均寻道长度
}
// SSTF算法
sstf(seq, n, m, direction) {
int move = 0; // 记录移动距离
int curr = m; // 记录当前磁头位置
bool visited[n];
memset(visited, false, sizeof(visited)); // 标记请求是否被服务过
for (int i = 0; i < n; i++) {
int min_dist = INT_MAX;
int min_idx = -1;
for (int j = 0; j < n; j++) {
if (!visited[j]) {
int dist = abs(curr - seq[j]);
if (dist < min_dist) {
min_dist = dist;
min_idx = j;
}
}
}
move += min_dist; // 计算移动距离
curr = seq[min_idx]; // 更新磁头位置
visited[min_idx] = true; // 标记请求已经被服务过
}
return move / n; // 返回平均寻道长度
}
// SCAN算法
scan(seq, n, m, direction) {
int move = 0; // 记录移动距离
int curr = m; // 记录当前磁头位置
int idx = -1; // 记录磁头移动方向
if (direction == 1) {
idx = 1;
} else {
idx = -1;
}
sort(seq, seq + n); // 将请求序列排序
int i;
for (i = 0; i < n; i++) {
if (seq[i] > curr) {
break;
}
}
if (i == n) {
i--;
idx = -1;
}
for (; i < n && i >= 0; i += idx) {
move += abs(curr - seq[i]); // 计算移动距离
curr = seq[i]; // 更新磁头位置
}
return move / n; // 返回平均寻道长度
}
// 循环SCAN算法
cscan(seq, n, m, direction) {
int move = 0; // 记录移动距离
int curr = m; // 记录当前磁头位置
int idx = -1; // 记录磁头移动方向
if (direction == 1) {
idx = 1;
} else {
idx = -1;
}
sort(seq, seq + n); // 将请求序列排序
int i;
for (i = 0; i < n; i++) {
if (seq[i] > curr) {
break;
}
}
if (i == n) {
i--;
idx = -1;
}
for (; i < n && i >= 0;) {
move += abs(curr - seq[i]); // 计算移动距离
curr = seq[i]; // 更新磁头位置
if (i == n - 1 && idx == 1) {
idx = -1;
} else if (i == 0 && idx == -1) {
idx = 1;
} else {
i += idx;
}
}
return move / n; // 返回平均寻道长度
}
```
这就是关于磁盘调度算法的解析和实现思路,希望对你有所帮助。
相关推荐
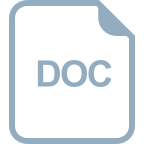
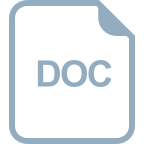
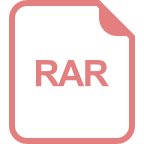













