batch_size = inputs.size(0)
时间: 2024-04-28 17:10:19 浏览: 9
batch_size = inputs.size(0)
This line of code determines the batch size of the input data. The input tensor is expected to have a shape of (batch_size, input_size), where batch_size is the number of samples in the batch and input_size is the number of input features. By calling the `size()` method on the input tensor and passing `0` as the argument, we obtain the size of the first dimension, which corresponds to the batch size. This value is then assigned to the `batch_size` variable.
相关问题
input_shape = (None, 24) inputs = Input(shape=input_shape) # 定义TCN网络的中间层,可以设置多个卷积层和池化层 tcn_layer = TCN(nb_filters=4, kernel_size=3, dilations=[1, 2, 4])(inputs) # 定义模型,将输入层和TCN网络的中间层连接起来 model = Model(inputs=inputs, outputs=tcn_layer) # 使用predict函数获取特征,将数据分成多个batch进行处理 num_samples = x_data.shape[0] features = [] batch_size = 32 for i in range(0, num_samples, batch_size): batch = x_data[i:i + batch_size] batch = tf.expand_dims(batch, axis=0) if i + batch_size > num_samples: batch_num = num_samples - i if batch_num == 1: feature_batch = model.predict(batch.reshape(1, *input_shape), batch_size=batch_num) else: feature_batch = model.predict(batch, batch_size=batch_num) features.append(feature_batch) else: feature_batch = model.predict(batch, batch_size=batch_size) features.append(feature_batch) features = np.concatenate(features, axis=0) print(features.shape)如果想要输出长度仍为x_data[0]
如果要在输出中保留原始数据 `x_data` 的第一个维度长度,你可以在预测结束后对 `features` 进行裁剪操作,使其第一个维度的长度与 `x_data` 的第一个维度长度相同。具体来说,你可以按照以下方式修改代码:
```python
import tensorflow as tf
# 定义输入层
input_shape = (None, 24)
inputs = Input(shape=input_shape)
# 定义 TCN 网络的中间层
tcn_layer = TCN(nb_filters=4, kernel_size=3, dilations=[1, 2, 4])(inputs)
# 定义模型
model = Model(inputs=inputs, outputs=tcn_layer)
# 使用 predict 函数获取特征,将数据分成多个 batch 进行处理
num_samples = x_data.shape[0]
features = []
batch_size = 32
for i in range(0, num_samples, batch_size):
batch = x_data[i:i + batch_size]
batch = tf.expand_dims(batch, axis=0)
if i + batch_size > num_samples:
batch_num = num_samples - i
if batch_num == 1:
feature_batch = model.predict(batch.reshape(1, *input_shape), batch_size=batch_num)
else:
feature_batch = model.predict(batch, batch_size=batch_num)
features.append(feature_batch)
else:
feature_batch = model.predict(batch, batch_size=batch_size)
features.append(feature_batch)
# 将 features 沿第一个维度拼接起来
features = np.concatenate(features, axis=0)
# 对 features 进行裁剪,使其第一个维度长度与 x_data 相同
features = features[:x_data.shape[0]]
print(features.shape)
```
在上面的代码中,我们在预测结束后对 `features` 进行了裁剪操作,使其第一个维度长度与 `x_data` 相同。这样就可以保留原始数据的第一个维度长度了。
定义输入数据的shape input_shape = (None, 24) # 定义模型的输入层 inputs = Input(shape=input_shape) # 定义TCN网络的中间层,可以设置多个卷积层和池化层 tcn_layer = TCN(nb_filters=4, kernel_size=3, dilations=[1, 2, 4, 8, 16])(inputs) # 定义模型,将输入层和TCN网络的中间层连接起来 model = Model(inputs=inputs, outputs=tcn_layer) # 使用predict函数获取特征,将数据分成多个batch进行处理 batch_size = 32 num_samples = train11s.shape[0] features = [] for i in range(0, num_samples, batch_size): batch = train11s[i:i + batch_size] if i + batch_size > num_samples: batch_size = num_samples - i if batch_size == 1: feature_batch = model.predict(batch.reshape(1, *input_shape), batch_size=batch_size) else: feature_batch = model.predict(batch, batch_size=batch_size) features.append(feature_batch) features = np.concatenate(features, axis=0) print(features.shape)出现错误
这段代码可能会出现错误,因为在循环中修改了batch_size的值。这可能会导致在处理最后一个batch时,batch_size的值小于32,从而导致维度不匹配的错误。建议将batch_size的值保存在另一个变量中,以避免这种错误。例如:
```
batch_size = 32
num_samples = train11s.shape[0]
features = []
for i in range(0, num_samples, batch_size):
batch = train11s[i:i + batch_size]
curr_batch_size = batch_size
if i + batch_size > num_samples:
curr_batch_size = num_samples - i
if curr_batch_size == 1:
feature_batch = model.predict(batch.reshape(1, *input_shape), batch_size=curr_batch_size)
else:
feature_batch = model.predict(batch, batch_size=curr_batch_size)
features.append(feature_batch)
features = np.concatenate(features, axis=0)
print(features.shape)
```
相关推荐
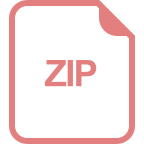
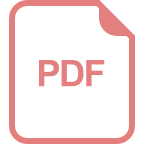













