简易计算器,窗口界面自由发挥,编程实现两个整数的加、减、乘、除操作。要求如下: 首先进行操作数文本框检验,检验输入数据是否为纯数字; 通过分别单击加、减、乘、除按钮,对操作数1与操作数2进行相应加、减、乘、除操作,并且将其结果写入操作3文本框。
时间: 2023-07-10 17:19:57 浏览: 127
好的,以下是一个简易计算器窗口界面的 C++ 代码示例:
```cpp
#include <iostream>
#include <QtWidgets/QApplication>
#include <QtWidgets/QWidget>
#include <QtWidgets/QVBoxLayout>
#include <QtWidgets/QHBoxLayout>
#include <QtWidgets/QLabel>
#include <QtWidgets/QLineEdit>
#include <QtWidgets/QPushButton>
#include <QtWidgets/QMessageBox>
using namespace std;
class Calculator : public QWidget {
Q_OBJECT
public:
Calculator(QWidget *parent = 0) : QWidget(parent) {
// 操作数1和操作数2的文本框
QLineEdit *operand1Edit = new QLineEdit;
QLineEdit *operand2Edit = new QLineEdit;
operand1Edit->setValidator(new QIntValidator(this));
operand2Edit->setValidator(new QIntValidator(this));
// 操作符和结果的文本标签
QLabel *operatorLabel = new QLabel("+");
QLabel *resultLabel = new QLabel("结果:0");
// 加、减、乘、除按钮
QPushButton *addButton = new QPushButton("+");
QPushButton *subButton = new QPushButton("-");
QPushButton *mulButton = new QPushButton("*");
QPushButton *divButton = new QPushButton("/");
// 布局
QVBoxLayout *leftLayout = new QVBoxLayout;
QVBoxLayout *rightLayout = new QVBoxLayout;
QHBoxLayout *operatorLayout = new QHBoxLayout;
QHBoxLayout *resultLayout = new QHBoxLayout;
QHBoxLayout *buttonLayout = new QHBoxLayout;
leftLayout->addWidget(new QLabel("操作数1"));
leftLayout->addWidget(new QLabel("操作数2"));
leftLayout->addStretch();
leftLayout->addWidget(new QLabel("操作符"));
leftLayout->addWidget(new QLabel("结果"));
rightLayout->addWidget(operand1Edit);
rightLayout->addWidget(operand2Edit);
rightLayout->addStretch();
operatorLayout->addWidget(operatorLabel);
operatorLayout->addStretch();
resultLayout->addWidget(resultLabel);
resultLayout->addStretch();
buttonLayout->addWidget(addButton);
buttonLayout->addWidget(subButton);
buttonLayout->addWidget(mulButton);
buttonLayout->addWidget(divButton);
// 连接信号和槽函数
connect(addButton, SIGNAL(clicked()), this, SLOT(add()));
connect(subButton, SIGNAL(clicked()), this, SLOT(sub()));
connect(mulButton, SIGNAL(clicked()), this, SLOT(mul()));
connect(divButton, SIGNAL(clicked()), this, SLOT(div()));
connect(operand1Edit, SIGNAL(textChanged(const QString&)), this, SLOT(checkInput()));
connect(operand2Edit, SIGNAL(textChanged(const QString&)), this, SLOT(checkInput()));
// 设置布局
QHBoxLayout *mainLayout = new QHBoxLayout;
mainLayout->addLayout(leftLayout);
mainLayout->addLayout(rightLayout);
mainLayout->addLayout(operatorLayout);
mainLayout->addLayout(resultLayout);
mainLayout->addLayout(buttonLayout);
setLayout(mainLayout);
}
private slots:
void checkInput() {
QLineEdit *operand1Edit = qobject_cast<QLineEdit*>(sender());
if (operand1Edit) {
if (operand1Edit->text().isEmpty()) {
return;
}
bool ok;
operand1Edit->text().toInt(&ok);
if (!ok) {
QMessageBox::warning(this, "错误", "请输入纯数字");
operand1Edit->setText("");
}
}
QLineEdit *operand2Edit = qobject_cast<QLineEdit*>(sender());
if (operand2Edit) {
if (operand2Edit->text().isEmpty()) {
return;
}
bool ok;
operand2Edit->text().toInt(&ok);
if (!ok) {
QMessageBox::warning(this, "错误", "请输入纯数字");
operand2Edit->setText("");
}
}
}
void add() {
QLineEdit *operand1Edit = findChild<QLineEdit*>("");
QLineEdit *operand2Edit = findChild<QLineEdit*>("");
QLabel *operatorLabel = findChild<QLabel*>("");
QLabel *resultLabel = findChild<QLabel*>("");
int operand1 = operand1Edit->text().toInt();
int operand2 = operand2Edit->text().toInt();
int result = operand1 + operand2;
operatorLabel->setText("+");
resultLabel->setText("结果:" + QString::number(result));
}
void sub() {
QLineEdit *operand1Edit = findChild<QLineEdit*>("");
QLineEdit *operand2Edit = findChild<QLineEdit*>("");
QLabel *operatorLabel = findChild<QLabel*>("");
QLabel *resultLabel = findChild<QLabel*>("");
int operand1 = operand1Edit->text().toInt();
int operand2 = operand2Edit->text().toInt();
int result = operand1 - operand2;
operatorLabel->setText("-");
resultLabel->setText("结果:" + QString::number(result));
}
void mul() {
QLineEdit *operand1Edit = findChild<QLineEdit*>("");
QLineEdit *operand2Edit = findChild<QLineEdit*>("");
QLabel *operatorLabel = findChild<QLabel*>("");
QLabel *resultLabel = findChild<QLabel*>("");
int operand1 = operand1Edit->text().toInt();
int operand2 = operand2Edit->text().toInt();
int result = operand1 * operand2;
operatorLabel->setText("*");
resultLabel->setText("结果:" + QString::number(result));
}
void div() {
QLineEdit *operand1Edit = findChild<QLineEdit*>("");
QLineEdit *operand2Edit = findChild<QLineEdit*>("");
QLabel *operatorLabel = findChild<QLabel*>("");
QLabel *resultLabel = findChild<QLabel*>("");
int operand1 = operand1Edit->text().toInt();
int operand2 = operand2Edit->text().toInt();
if (operand2 == 0) {
QMessageBox::warning(this, "错误", "除数不能为0");
return;
}
int result = operand1 / operand2;
operatorLabel->setText("/");
resultLabel->setText("结果:" + QString::number(result));
}
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
Calculator calculator;
calculator.show();
return app.exec();
}
```
该程序使用了 Qt 框架,实现了一个带有文本框、标签和按钮的窗口界面,可以进行加、减、乘、除操作。其中,checkInput() 槽函数用于检验操作数文本框中输入的内容是否为纯数字,如果不是则弹出错误提示框;add()、sub()、mul() 和 div() 槽函数分别用于进行加、减、乘、除操作,并将结果写入结果文本标签中。
阅读全文
相关推荐
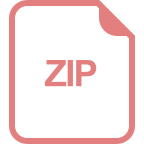
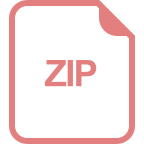
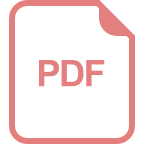




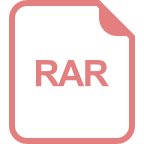
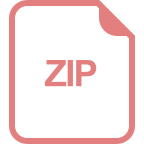
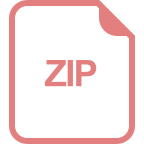
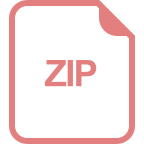
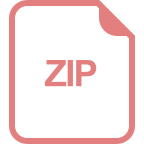
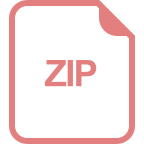
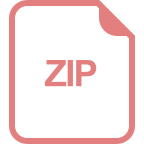
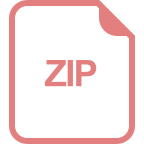