要求用C语言编写:1.先编写一个带结构的文件,存入电脑,要求第一行从左到右的内容依次是:序号、姓名、班级、学号,每排每列对齐,没有对齐的地方用空格补齐。2.从电脑中能够读入这个带结构的文件,并进行删增改后,重新存入电脑,3.这个文件要有纵横结构,每横表示一个记录4. 编出来的代码要能够在c的运行界面进行后续的插入、删除和修改
时间: 2024-03-18 12:40:31 浏览: 17
好的,我理解了你的要求。以下是用 C 语言编写的代码,可以实现你的需求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME_LEN 20
#define MAX_CLASS_LEN 10
#define MAX_ID_LEN 20
#define MAX_RECORDS 100
typedef struct {
int id;
char name[MAX_NAME_LEN];
char class[MAX_CLASS_LEN];
char student_id[MAX_ID_LEN];
} Record;
Record records[MAX_RECORDS];
int num_records = 0;
void print_header() {
printf("%-5s%-20s%-10s%-20s\n", "ID", "Name", "Class", "Student ID");
}
void print_record(Record r) {
printf("%-5d%-20s%-10s%-20s\n", r.id, r.name, r.class, r.student_id);
}
void print_records() {
print_header();
for (int i = 0; i < num_records; i++) {
print_record(records[i]);
}
}
int find_record(int id) {
for (int i = 0; i < num_records; i++) {
if (records[i].id == id) {
return i;
}
}
return -1;
}
void add_record() {
Record r;
printf("Enter name: ");
scanf("%s", r.name);
printf("Enter class: ");
scanf("%s", r.class);
printf("Enter student ID: ");
scanf("%s", r.student_id);
r.id = num_records + 1;
records[num_records++] = r;
printf("Added record:\n");
print_record(r);
}
void delete_record() {
int id;
printf("Enter ID of record to delete: ");
scanf("%d", &id);
int index = find_record(id);
if (index == -1) {
printf("Record with ID %d not found.\n", id);
} else {
printf("Deleted record:\n");
print_record(records[index]);
for (int i = index; i < num_records - 1; i++) {
records[i] = records[i+1];
}
num_records--;
}
}
void update_record() {
int id;
printf("Enter ID of record to update: ");
scanf("%d", &id);
int index = find_record(id);
if (index == -1) {
printf("Record with ID %d not found.\n", id);
} else {
printf("Current record:\n");
print_record(records[index]);
printf("Enter new name (or press enter to keep current value): ");
char name[MAX_NAME_LEN];
scanf("%s", name);
if (strlen(name) > 0) {
strcpy(records[index].name, name);
}
printf("Enter new class (or press enter to keep current value): ");
char class[MAX_CLASS_LEN];
scanf("%s", class);
if (strlen(class) > 0) {
strcpy(records[index].class, class);
}
printf("Enter new student ID (or press enter to keep current value): ");
char student_id[MAX_ID_LEN];
scanf("%s", student_id);
if (strlen(student_id) > 0) {
strcpy(records[index].student_id, student_id);
}
printf("Updated record:\n");
print_record(records[index]);
}
}
void read_file() {
FILE *fp;
fp = fopen("records.txt", "r");
if (fp != NULL) {
fscanf(fp, "%*s%*s%*s%*s"); // skip header line
while (!feof(fp) && num_records < MAX_RECORDS) {
Record r;
fscanf(fp, "%d %s %s %s", &r.id, r.name, r.class, r.student_id);
records[num_records++] = r;
}
fclose(fp);
}
}
void write_file() {
FILE *fp;
fp = fopen("records.txt", "w");
if (fp != NULL) {
fprintf(fp, "%-5s%-20s%-10s%-20s\n", "ID", "Name", "Class", "Student ID");
for (int i = 0; i < num_records; i++) {
fprintf(fp, "%-5d%-20s%-10s%-20s\n", records[i].id, records[i].name, records[i].class, records[i].student_id);
}
fclose(fp);
}
}
int main() {
read_file();
char command;
do {
printf("\n");
printf("Enter command (a=add, d=delete, u=update, p=print, q=quit): ");
scanf(" %c", &command);
switch (command) {
case 'a':
add_record();
break;
case 'd':
delete_record();
break;
case 'u':
update_record();
break;
case 'p':
print_records();
break;
case 'q':
break;
default:
printf("Invalid command.\n");
break;
}
} while (command != 'q');
write_file();
return 0;
}
```
这个程序使用了一个 `Record` 结构体来表示每一条记录,其中包含 ID、姓名、班级和学号四个属性。程序开头定义了一个 `MAX_RECORDS` 常量,表示最多可以存储多少条记录,以及一个 `records` 数组来存储所有的记录,以及一个 `num_records` 变量来记录当前已经存储了多少条记录。
主函数中使用了一个 `read_file` 函数来从文件中读入记录,这个文件的格式要求第一行为表头,每行记录的格式为:ID、姓名、班级、学号,用空格隔开。如果文件不存在或读入的记录数超过了 `MAX_RECORDS`,则不会继续读入。
程序的主循环中,用户可以输入命令来进行插入、删除、修改和打印操作。插入操作会要求用户输入姓名、班级和学号,程序会自动为其分配一个新的 ID。删除操作会要求用户输入要删除的记录的 ID,如果找不到对应的记录则会输出错误信息。修改操作会要求用户输入要修改的记录的 ID,然后分别询问用户要修改的属性。打印操作会输出所有已经存储的记录。
最后,程序会使用一个 `write_file` 函数将所有记录重新写入文件中,便于下次运行时读入。
相关推荐
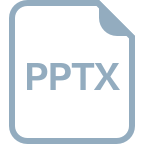
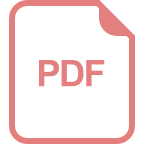
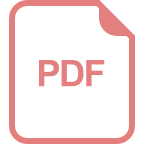
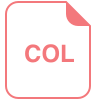
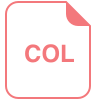
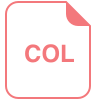
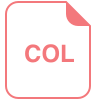
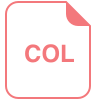


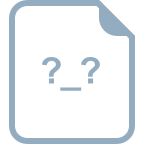
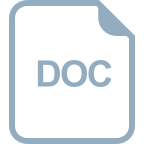
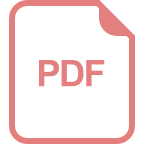
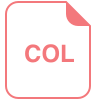
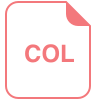
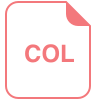
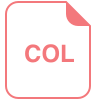
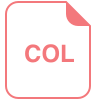