c++去掉字符串中的\n
时间: 2024-05-04 09:21:51 浏览: 219
在 C++ 中,可以使用字符串的 erase 和 remove 函数来去除字符串中的换行符。具体步骤如下:
1. 使用 find 函数找到字符串中的换行符位置。
2. 使用 erase 函数删除该位置处的字符。
3. 重复步骤 1 和 2 直到字符串中不存在换行符为止。
示例代码如下:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "hello\nworld\n";
size_t pos = 0;
while ((pos = str.find('\n', pos)) != string::npos) {
str.erase(pos, 1);
}
cout << str << endl; // 输出 hello world
return 0;
}
```
在上面的代码中,我们使用了 while 循环来反复查找和删除字符串中的换行符。在每次循环中,find 函数会返回字符串中第一个换行符的位置,如果返回值等于 string::npos,则表示已经没有换行符了,循环结束。如果找到了换行符,就使用 erase 函数删除该位置处的字符。注意,erase 函数的第二个参数是要删除的字符个数,这里只删除一个字符。最后,输出处理后的字符串即可。
相关问题
c++ 去掉字符串中的\n
可以使用C++的字符串处理函数erase和find来去掉字符串中的\n,具体代码如下:
```c++
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str = "hello\nworld\n";
size_t pos = 0;
while ((pos = str.find("\n", pos)) != string::npos) {
str.erase(pos, 1);
}
cout << str << endl;
return 0;
}
```
运行结果为:
```
helloworld
```
C++去掉字符串数组中的\n
可以使用C++中的字符串处理函数`std::string`的`erase`函数和`std::remove`函数来去掉字符串数组中的`\n`。
示例代码:
```c++
#include <iostream>
#include <string>
#include <algorithm>
int main() {
char str[] = "hello\nworld\n";
std::string s(str);
// 使用 erase 和 remove 去掉 \n
s.erase(std::remove(s.begin(), s.end(), '\n'), s.end());
std::cout << s << std::endl;
return 0;
}
```
输出:
```
helloworld
```
阅读全文
相关推荐
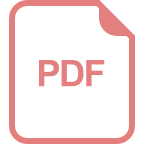
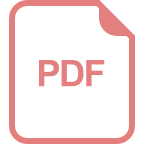
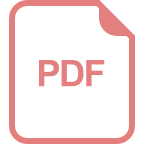













