1.1编写程序,建立2个带头结点单链表,输入若干整数将正整数插入第1个单链表,将负整数插入第2个单链表,插入前和插入后单链表保持递增或相等次序,显示2个单链表,最后销毁。程序不可存在内存泄漏。
时间: 2024-03-23 14:27:48 浏览: 31
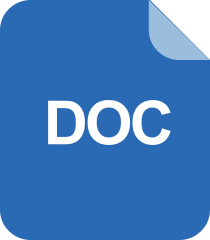
链表,建立链表、遍历链表、排序、去重、反转。。。。

#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *next;
} Node, *LinkedList;
void initList(LinkedList *L) {
*L = (LinkedList)malloc(sizeof(Node));
(*L)->next = NULL;
}
void insert(LinkedList L, int x) {
Node *p = L;
while (p->next && p->next->data < x) {
p = p->next;
}
Node *node = (Node*)malloc(sizeof(Node));
node->data = x;
node->next = p->next;
p->next = node;
}
void printList(LinkedList L) {
Node *p = L->next;
while (p) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
void destroyList(LinkedList *L) {
Node *p = (*L)->next;
while (p) {
Node *q = p;
p = p->next;
free(q);
}
free(*L);
*L = NULL;
}
int main() {
LinkedList L1, L2;
initList(&L1);
initList(&L2);
int x;
while (scanf("%d", &x) != EOF) {
if (x > 0) {
insert(L1, x);
} else {
insert(L2, x);
}
}
printList(L1);
printList(L2);
destroyList(&L1);
destroyList(&L2);
return 0;
}
阅读全文
相关推荐
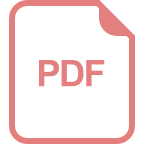
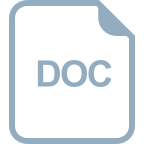







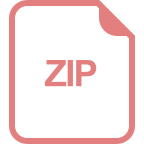
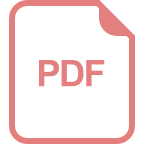
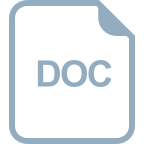
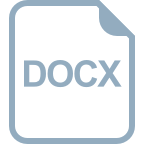
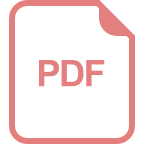
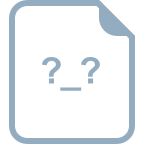
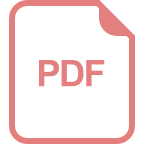