uni-app写一个领券界面
时间: 2023-07-07 19:07:37 浏览: 116
好的,下面是一个简单的领券界面示例,包括优惠券列表、领取按钮和弹窗提示:
1. 在 uni-app 中创建一个页面,比如命名为 coupon.vue。
2. 在 coupon.vue 中添加以下代码:
```html
<template>
<view class="container">
<view class="coupon-list">
<view class="coupon" v-for="(coupon, index) in couponList" :key="index">
<view class="coupon-info">
<view class="coupon-name">{{ coupon.name }}</view>
<view class="coupon-desc">{{ coupon.desc }}</view>
<view class="coupon-time">{{ coupon.time }}</view>
</view>
<view class="coupon-receive">
<button class="coupon-btn" @click="showPopup(index)">领取</button>
</view>
</view>
</view>
<van-popup v-model="showPopup" position="bottom">
<view class="popup-content">
<view class="popup-title">{{ currentCoupon.name }}</view>
<view class="popup-desc">{{ currentCoupon.desc }}</view>
<view class="popup-time">{{ currentCoupon.time }}</view>
<button class="popup-btn" @click="receiveCoupon">立即领取</button>
</view>
</van-popup>
</view>
</template>
<script>
export default {
data() {
return {
couponList: [
{
name: '优惠券1',
desc: '满100减50',
time: '有效期:2021-12-31'
},
{
name: '优惠券2',
desc: '满200减100',
time: '有效期:2021-12-31'
},
{
name: '优惠券3',
desc: '满300减150',
time: '有效期:2021-12-31'
}
],
showPopup: false,
currentCoupon: {}
}
},
methods: {
showPopup(index) {
this.currentCoupon = this.couponList[index];
this.showPopup = true;
},
receiveCoupon() {
// 领取优惠券的逻辑,可以通过接口请求实现
this.showPopup = false;
uni.showToast({
title: '领取成功',
icon: 'success'
});
}
}
}
</script>
<style>
.container {
padding: 20rpx;
}
.coupon-list {
margin-top: 20rpx;
}
.coupon {
display: flex;
justify-content: space-between;
align-items: center;
margin-bottom: 20rpx;
padding: 20rpx;
border: 1px solid #eee;
border-radius: 10rpx;
}
.coupon-info {
flex: 1;
margin-right: 20rpx;
}
.coupon-name {
font-size: 36rpx;
font-weight: bold;
margin-bottom: 10rpx;
}
.coupon-desc {
color: #888;
font-size: 28rpx;
margin-bottom: 10rpx;
}
.coupon-time {
color: #888;
font-size: 28rpx;
}
.coupon-receive {
width: 200rpx;
}
.coupon-btn {
width: 100%;
height: 60rpx;
line-height: 60rpx;
text-align: center;
background-color: #f44;
color: #fff;
border-radius: 10rpx;
}
.popup-content {
padding: 20rpx;
}
.popup-title {
font-size: 36rpx;
font-weight: bold;
margin-bottom: 10rpx;
}
.popup-desc {
color: #888;
font-size: 28rpx;
margin-bottom: 10rpx;
}
.popup-time {
color: #888;
font-size: 28rpx;
}
.popup-btn {
width: 100%;
height: 60rpx;
line-height: 60rpx;
text-align: center;
background-color: #f44;
color: #fff;
border-radius: 10rpx;
}
</style>
```
3. 这段代码定义了一个 coupon 组件,包含一个优惠券列表和一个弹窗提示。couponList 数组存储了三个优惠券的基本信息,showPopup 变量控制弹窗的显示和隐藏,currentCoupon 变量存储当前选中的优惠券信息。
4. 优惠券列表中的每个优惠券都可以点击领取按钮,调用 showPopup 方法弹出提示框。弹出框中展示当前优惠券的详细信息,点击立即领取按钮可以执行领取优惠券的逻辑。
5. 最后,在 coupon.vue 中引入 vant-ui 库,因为示例中用到了 vant-popup 组件。可以通过以下命令安装 vant-ui:
```bash
npm install vant-weapp -S --production
```
6. 在 coupon.vue 中引入 vant-ui:
```javascript
import { Popup } from 'vant-weapp';
export default {
components: {
[Popup.name]: Popup
},
// ...
}
```
7. 最后,运行 uni-app,即可看到一个简单的领券界面。
相关推荐
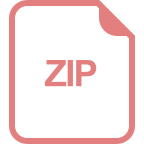
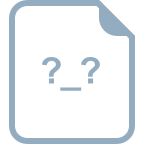














