使用python完成一个程序,实现功能,获取给定字符的编码
时间: 2024-05-07 09:17:44 浏览: 10
好的,可以使用Python内置的ord()函数获取字符的Unicode编码,然后使用hex()函数将其转换为16进制编码。下面是一个简单的示例代码:
```
char = 'A'
unicode_code = ord(char)
hex_code = hex(unicode_code)
print(f"The Unicode code of '{char}' is {unicode_code}.")
print(f"The hex code of '{char}' is {hex_code}.")
```
输出结果:
```
The Unicode code of 'A' is 65.
The hex code of 'A' is 0x41.
```
以上是一个简单的实现,您可以根据需要进行修改和完善。
相关问题
使用Python实现哈夫曼编码
哈夫曼编码是一种无损压缩算法,通过使用变长编码来表示不同的字符,使得频繁出现的字符使用较短的编码,从而减小了数据的存储空间。以下是使用 Python 实现哈夫曼编码的代码示例:
```python
import heapq
from collections import defaultdict
class Node:
def __init__(self, freq, char=None):
self.freq = freq
self.char = char
self.left = None
self.right = None
def __lt__(self, other):
return self.freq < other.freq
def build_huffman_tree(text):
freq_dict = defaultdict(int)
for char in text:
freq_dict[char] += 1
heap = []
for char, freq in freq_dict.items():
heapq.heappush(heap, Node(freq, char))
while len(heap) > 1:
left = heapq.heappop(heap)
right = heapq.heappop(heap)
parent = Node(left.freq + right.freq)
parent.left = left
parent.right = right
heapq.heappush(heap, parent)
return heap[0]
def build_huffman_table(node, code='', table={}):
if node.char:
table[node.char] = code
else:
build_huffman_table(node.left, code + '0', table)
build_huffman_table(node.right, code + '1', table)
return table
def encode(text):
huffman_tree = build_huffman_tree(text)
huffman_table = build_huffman_table(huffman_tree)
encoded_text = ''
for char in text:
encoded_text += huffman_table[char]
return encoded_text
def decode(encoded_text, huffman_tree):
decoded_text = ''
node = huffman_tree
for bit in encoded_text:
if bit == '0':
node = node.left
else:
node = node.right
if node.char:
decoded_text += node.char
node = huffman_tree
return decoded_text
if __name__ == '__main__':
text = 'hello world'
encoded_text = encode(text)
print('Encoded Text:', encoded_text)
huffman_tree = build_huffman_tree(text)
decoded_text = decode(encoded_text, huffman_tree)
print('Decoded Text:', decoded_text)
```
该代码中的 `build_huffman_tree` 函数使用给定的文本构建哈夫曼树,并返回根节点。`build_huffman_table` 函数使用构建好的哈夫曼树生成编码表,编码表以字典的形式返回。`encode` 函数使用编码表将文本编码为哈夫曼编码,并返回编码后的字符串。`decode` 函数使用给定的哈夫曼树将编码的字符串解码为原始文本,并返回解码后的字符串。
给定一个经过编码的字符串,返回它解码后的字符串
这是一个经典的字符串解码问题,可以使用栈来实现。
算法步骤如下:
1. 初始化一个栈,并将初始字符串设为当前字符串
2. 从左到右遍历字符串中的每个字符:
a. 如果当前字符是数字,解析出完整的数字并将其压入栈中
b. 如果当前字符是字母,直接将其压入栈中
c. 如果当前字符是左括号,将当前字符串和栈顶元素压入栈中,并将当前字符串重置为空字符串
d. 如果当前字符是右括号,弹出栈顶的字符串和数字,重复数字次数后将弹出的字符串拼接到当前字符串的末尾
3. 返回当前字符串
Python实现代码如下:
```python
def decodeString(s: str) -> str:
stack = []
cur_str = ""
cur_num = 0
for c in s:
if c.isdigit():
cur_num = cur_num * 10 + int(c)
elif c.isalpha():
cur_str += c
elif c == "[":
stack.append(cur_str)
stack.append(cur_num)
cur_str = ""
cur_num = 0
elif c == "]":
num = stack.pop()
prev_str = stack.pop()
cur_str = prev_str + num * cur_str
return cur_str
```
例如,给定字符串 "3[a]2[bc]",解码后得到 "aaabcbc"。
相关推荐
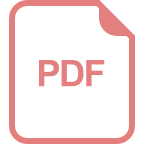
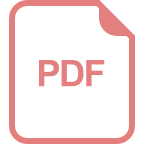
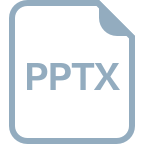












