每个学生包含姓名、性别、学号、成绩的输入,至少输入10个学生,并将成绩 按照男女分离形成两个单链表,并对两个单链表进行排序。
时间: 2024-03-11 14:43:26 浏览: 55
好的,这是一个比较完整的题目,需要包含输入、链表分离、排序等多个步骤。以下是一个示例的C++代码实现,希望能对你有所帮助:
```cpp
#include <iostream>
#include <string>
using namespace std;
struct Student {
string name;
string gender;
string id;
int score;
Student* next;
};
void printList(Student* head) {
Student* curr = head;
while (curr != nullptr) {
cout << curr->name << " " << curr->gender << " " << curr->id << " " << curr->score << endl;
curr = curr->next;
}
}
void separateList(Student* head, Student*& maleHead, Student*& femaleHead) {
Student* curr = head;
while (curr != nullptr) {
Student* next = curr->next;
if (curr->gender == "male") {
curr->next = maleHead;
maleHead = curr;
} else {
curr->next = femaleHead;
femaleHead = curr;
}
curr = next;
}
}
void sortList(Student*& head) {
if (head == nullptr || head->next == nullptr) {
return;
}
Student* prev = nullptr;
Student* curr = head;
while (curr != nullptr) {
Student* next = curr->next;
Student* temp = head;
Student* tempPrev = nullptr;
while (temp != curr && temp->score > curr->score) {
tempPrev = temp;
temp = temp->next;
}
if (temp != curr) {
if (tempPrev == nullptr) {
head = curr;
} else {
tempPrev->next = curr;
}
curr->next = temp;
prev->next = next;
} else {
prev = curr;
}
curr = next;
}
}
int main() {
Student* head = nullptr;
for (int i = 0; i < 10; i++) {
string name, gender, id;
int score;
cin >> name >> gender >> id >> score;
Student* student = new Student();
student->name = name;
student->gender = gender;
student->id = id;
student->score = score;
student->next = head;
head = student;
}
Student* maleHead = nullptr;
Student* femaleHead = nullptr;
separateList(head, maleHead, femaleHead);
cout << "Male students:" << endl;
printList(maleHead);
cout << "Female students:" << endl;
printList(femaleHead);
sortList(maleHead);
sortList(femaleHead);
cout << "Sorted male students:" << endl;
printList(maleHead);
cout << "Sorted female students:" << endl;
printList(femaleHead);
return 0;
}
```
在这个例子中,我们首先定义了一个名为 `Student` 的结构体,用于存储每个学生的姓名、性别、学号和成绩,以及指向下一个学生的指针。然后,我们使用一个 `for` 循环来读取10个学生的信息,并将每个学生作为一个节点添加到链表中。接着,我们使用 `separateList` 函数将链表按照男女分离形成两个单链表,具体实现方式是遍历原链表,根据每个学生的性别将其添加到对应的单链表中。最后,我们使用 `sortList` 函数对两个单链表进行排序,具体实现方式是插入排序。最后,我们使用 `printList` 函数输出排序后的两个单链表。
阅读全文
相关推荐
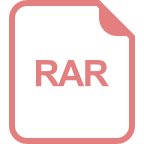
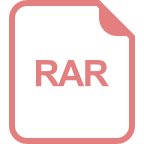















