c语言多线程
时间: 2023-06-21 16:20:13 浏览: 74
C语言中的多线程可以通过使用线程库来实现,比如POSIX线程库(pthread)和Windows线程库。以下是一个简单的例子,展示如何使用pthread库创建和使用线程:
```c
#include <stdio.h>
#include <pthread.h>
void *my_thread(void *arg) {
int thread_index = *(int *)arg;
printf("This is thread #%d\n", thread_index);
pthread_exit(NULL);
}
int main() {
pthread_t threads[5];
int thread_args[5];
// 创建5个线程
for (int i = 0; i < 5; i++) {
thread_args[i] = i;
pthread_create(&threads[i], NULL, my_thread, (void *)&thread_args[i]);
}
// 等待线程结束
for (int i = 0; i < 5; i++) {
pthread_join(threads[i], NULL);
}
printf("All threads have finished execution\n");
return 0;
}
```
在上述代码中,我们使用了pthread库创建了5个线程,并在每个线程中输出了线程编号。在主函数中,我们使用pthread_join函数等待所有线程结束后再输出一条信息。
相关推荐
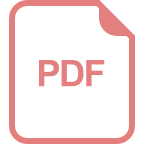
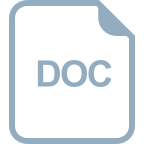
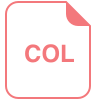
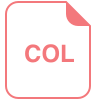
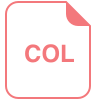
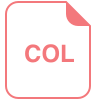
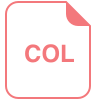









