c# mongodb convert between model and bson
时间: 2023-10-19 13:22:20 浏览: 43
To convert between a model and BSON in C# with MongoDB, you can use the `BsonDocument` class and the `BsonSerializer` class from the MongoDB C# driver.
To convert a model to BSON:
```
using MongoDB.Bson;
using MongoDB.Bson.Serialization;
public class Person
{
public ObjectId Id { get; set; }
public string Name { get; set; }
public int Age { get; set; }
}
Person person = new Person { Name = "John", Age = 30 };
BsonDocument bsonDocument = person.ToBsonDocument();
```
To convert BSON to a model:
```
using MongoDB.Bson;
using MongoDB.Bson.Serialization;
BsonDocument bsonDocument = ... // retrieve the document from MongoDB
Person person = BsonSerializer.Deserialize<Person>(bsonDocument);
```
Note that you should replace the `...` with the code that retrieves the document from MongoDB.
If you need to convert a list of models to BSON, you can use `BsonArray` and `ToBsonDocumentArray`:
```
List<Person> people = new List<Person>
{
new Person { Name = "John", Age = 30 },
new Person { Name = "Jane", Age = 25 }
};
BsonArray bsonArray = new BsonArray(people.Select(x => x.ToBsonDocument()));
BsonDocument bsonDocument = new BsonDocument { { "people", bsonArray } };
```
And to convert BSON to a list of models:
```
BsonDocument bsonDocument = ... // retrieve the document from MongoDB
BsonArray bsonArray = bsonDocument["people"].AsBsonArray;
List<Person> people = bsonArray.Select(x => BsonSerializer.Deserialize<Person>(x.AsBsonDocument)).ToList();
```
Again, you should replace the `...` with the code that retrieves the document from MongoDB.
相关推荐
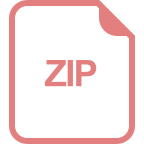
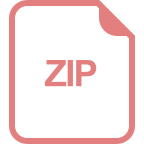














