这个注解是/** * Mapped onto a entity bean property that represents the user id of * who created the entity> * <p> * To use this annotation you need to implement CurrentUserProvider. * The type of the bean property should match the type returned by * CurrentUserProvider. * </p> * <h3>Example:</h3> * <pre>{@code * * @WhoCreated * String whoCreated; * * }</pre> */ @Target({ElementType.FIELD}) @Retention(RetentionPolicy.RUNTIME) public @interface WhoCreated { }
时间: 2023-11-12 21:06:46 浏览: 141
根据您提供的代码,`@WhoCreated` 是一个自定义注解,用于标记实体类中表示创建者的属性。根据注解的描述,它需要与 `CurrentUserProvider` 接口一起使用,并且被标记的属性的类型应该与 `CurrentUserProvider` 返回的类型相匹配。
以下是使用 `@WhoCreated` 注解的示例代码:
```java
public class MyEntity {
// 其他字段...
@WhoCreated
private String whoCreated;
// 其他方法...
}
```
在上述示例中,`whoCreated` 属性被标记为 `@WhoCreated` 注解,表示它是用于保存创建者信息的字段。
要正确使用 `@WhoCreated` 注解,您需要实现一个 `CurrentUserProvider` 接口,并确保返回的类型与被标记属性的类型相匹配。`CurrentUserProvider` 接口负责提供当前用户的信息,以便在创建实体对象时将其赋值给被标记的属性。
请注意,以上代码只是示例,并且假设您已经实现了 `CurrentUserProvider` 接口。实际情况可能因您所使用的框架或库而有所不同。
如果您需要进一步的帮助,请提供有关 `CurrentUserProvider` 接口的细节或相关代码的信息,我将尽力协助您解决问题。
相关问题
You are creating a Web application for the book shop by using Spring MVC. When a user sends a request for theURL, /SearchBook.htm page in this Web application, the request needs to be handled by theSearchBookController class defined in the controller package of the Web application. For this, you used thefollowing code snippet:<beans> <bean id="ControllerHandlerMapping" class=org.springframework.web.servlet.mvcsupport,SimpleUrlHandlerMapping"/><bean class="controller SearchBookController"></beans> However, the preceding code snippet does not forward the request to the SearchBookController class. Analyze theproblem and provide the correct code snippet.
The code snippet provided in the question is incorrect because the `SimpleUrlHandlerMapping` bean is not properly configured to handle the URL mapping for the `SearchBookController`. To correctly map the URL `/SearchBook.htm` to the `SearchBookController`, you should use the following code snippet:
```xml
<beans>
<bean id="handlerMapping" class="org.springframework.web.servlet.handler.SimpleUrlHandlerMapping">
<property name="mappings">
<props>
<prop key="/SearchBook.htm">searchBookController</prop>
</props>
</property>
</bean>
<bean id="searchBookController" class="controller.SearchBookController"/>
</beans>
```
In this code snippet, we are using the `SimpleUrlHandlerMapping` bean to map the URL `/SearchBook.htm` to the `searchBookController` bean, which is an instance of the `SearchBookController` class. The `handlerMapping` bean is configured with a `mappings` property that contains a `props` element with a single URL mapping for `/SearchBook.htm`. The `searchBookController` bean is defined as an instance of the `SearchBookController` class and is mapped to the `searchBookController` bean ID. This configuration ensures that requests to the `/SearchBook.htm` URL are handled by the `SearchBookController` class.
@Data @AllArgsConstructor @NoArgsConstructor @Builder @Table(name = "media_file") public class MediaFile { /** * 主键 */ @Id @GeneratedValue(strategy = GenerationType.IDENTITY) @Column(name = "id") private Integer id; /** * 媒体文件名称 */ @Column(name = "name") @ApiModelProperty(value = "媒体文件名称") private String fileName; /** * 媒体文件类型:0-图片,1-视频 */ @Column(name = "type") @ApiModelProperty(value = "媒体文件类型") private Integer fileType; /** * 媒体文件URL地址 */ @Column(name = "url") @ApiModelProperty(value = "媒体文件URL地址") private String fileUrl; /** * 创建时间 */ @Column(name = "create_time") @ApiModelProperty(value = "创建时间") private Date createTime; /** * 更新时间 */ @Column(name = "update_time") @ApiModelProperty(value = "更新时间") private Date updateTime; @OneToMany(mappedBy = "mediaFile", cascade = CascadeType.ALL) private List<Advert> adverts = new ArrayList<>(); }与@Insert("insert into media_file(name, type, url) values (#{fileName}, #{fileType}, #{fileUrl})") @SelectKey(statement = "select last_insert_id()", keyProperty = "id", before = false, resultType = int.class) int insertAndGetId(MediaFile mediaFile);没有正确返回id
根据提供的代码,可能存在以下原因导致没有正确返回id:
1. 在插入数据时,没有设置id字段的值,而是使用了自增主键,因此需要在插入数据后使用@SelectKey来获取自增的id值。但是,可能存在无法正确获取自增id的情况,可以尝试检查数据库的设置是否正确或者修改@SelectKey的参数。
2. 在调用insertAndGetId方法时,没有正确传入MediaFile对象,导致无法正确插入数据或者获取id。可以尝试检查调用insertAndGetId方法时传入的MediaFile对象是否正确。
3. 在插入数据时,存在重复的name、type和url组合,导致无法插入或者获取id。可以尝试检查数据库中是否存在重复的数据记录。
阅读全文
相关推荐
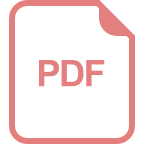
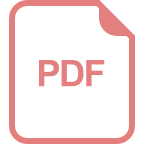
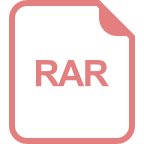
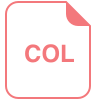













