使用c语言编写程序:编写函数,将两个字符串s和t连接起来。s、t的长度均不超过100。不得使用系统库函数。 若s为FirstString--,t为SecondString,则输出结果为FirstString--SecondString。第一行为测试数据的组数T,后跟T组测试数据,每组测试数据包含两行,第一行为字符串s,第二行为字符串t。对于每组测试数据,输出结果单独占一行,为连接后的新字符串。
时间: 2024-02-24 15:54:35 浏览: 17
以下是使用 C 语言编写的程序,实现将两个字符串 s 和 t 连接起来的功能:
```c
#include <stdio.h>
void str_concat(char *s, char *t)
{
while (*s != '\0') { // 找到 s 的末尾
s++;
}
while (*t != '\0') { // 将 t 连接到 s 的末尾
*s = *t;
s++;
t++;
}
*s = '\0'; // 结尾字符
}
int main()
{
int T;
scanf("%d", &T); // 输入测试数据组数
while (T--) {
char s[101], t[101];
scanf("%s%s", s, t); // 输入两个字符串
str_concat(s, t); // 将两个字符串连接起来
printf("%s\n", s); // 输出新字符串
}
return 0;
}
```
输入格式为:
```
T
s1
t1
s2
t2
...
```
其中,T 表示测试数据组数,si 和 ti 分别表示两个字符串。输出格式为:
```
s1t1
s2t2
...
```
每个新字符串单独占一行。
相关问题
用c语言编写:将两个字符串连接起来,不使用stract()函数
可以使用指针操作和循环来将两个字符串连接起来。以下是一个简单的示例程序,将字符串s1和s2连接起来:
```c
#include <stdio.h>
int main()
{
char s1[100], s2[100];
int i, j;
printf("Enter the first string: ");
scanf("%s", s1);
printf("Enter the second string: ");
scanf("%s", s2);
// Find the end of the first string
i = 0;
while (s1[i] != '\0') {
i++;
}
// Append the second string to the first string
j = 0;
while (s2[j] != '\0') {
s1[i] = s2[j];
i++;
j++;
}
// Terminate the string with a null character
s1[i] = '\0';
printf("The concatenated string is: %s\n", s1);
return 0;
}
```
在此示例程序中,我们首先使用scanf()函数从用户输入中读取两个字符串s1和s2。然后,我们使用一个while循环找到字符串s1的末尾(即null字符'\0'之前的最后一个字符的索引)。然后,我们使用另一个while循环将字符串s2附加到字符串s1的末尾。最后,我们在新字符串的末尾添加了一个null字符,以将其作为C字符串的终止符。
请注意,这只是一个简单的示例,可能会有一些边缘情况需要处理(例如,如果输入的字符串长度超过了s1和s2数组的大小)。
使用c语言编写函数,将两个字符串s和t连接起来。s、t的长度均不超过100。不得使用系统库函数。 若s为FirstString--,t为SecondString,则输出结果为FirstString--SecondString。
可以使用指针和循环来实现字符串连接的功能,可以按照以下方法来编写函数:
```c
void string_concat(char *s, char *t) {
// 找到s的末尾
while (*s != '\0') {
s++;
}
// 将t复制到s后面
while (*t != '\0') {
*s = *t;
s++;
t++;
}
// 添加字符串结束标志
*s = '\0';
}
```
在上述代码中,第一个while循环用于找到字符串s的末尾,第二个while循环用于将字符串t复制到s的末尾,最后添加字符串结束标志'\0'。可以在main函数中调用该函数实现字符串连接的功能:
```c
#include <stdio.h>
void string_concat(char *s, char *t);
int main() {
char s[101] = "FirstString--";
char t[101] = "SecondString";
string_concat(s, t);
printf("%s\n", s); // 输出结果为FirstString--SecondString
return 0;
}
void string_concat(char *s, char *t) {
// 找到s的末尾
while (*s != '\0') {
s++;
}
// 将t复制到s后面
while (*t != '\0') {
*s = *t;
s++;
t++;
}
// 添加字符串结束标志
*s = '\0';
}
```
注意,为了确保字符串s有足够的空间来存储连接后的字符串,需要保证字符串s的长度不小于两个字符串长度之和。
相关推荐
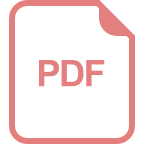
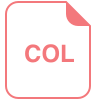
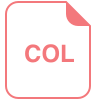
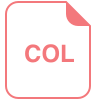
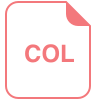
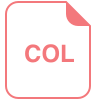







