编写一个函数,将两个字符串连接。只能用c语言
时间: 2023-06-24 17:03:06 浏览: 127
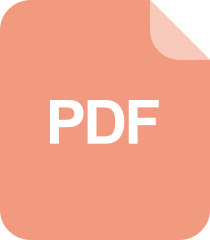
C语言 不使用strcat函数实现连接两个字符串功能代码
可以使用C语言中的字符串拼接函数`strcat()`来实现两个字符串的连接。
示例代码如下:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str1[100] = "Hello, ";
char str2[] = "world!";
strcat(str1, str2); // 将str2连接到str1后面
printf("连接后的字符串为:%s\n", str1);
return 0;
}
```
输出结果为:
```
连接后的字符串为:Hello, world!
```
可以看到,通过`strcat()`函数,我们把`str2`字符串连接到了`str1`字符串的末尾。注意,`strcat()`函数会改变原来的字符串,因此在使用时需要注意字符串的长度,以避免发生内存溢出等错误。
阅读全文
相关推荐
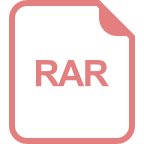


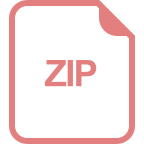
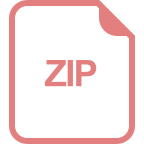
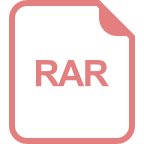
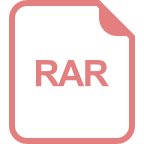
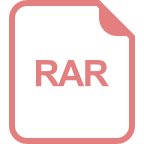
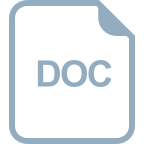
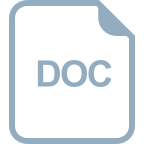

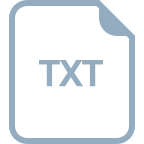
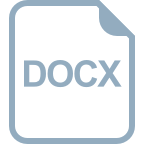
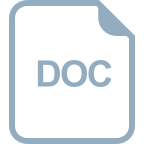