python的openpyxl遍历sheet
时间: 2023-04-23 08:07:31 浏览: 448
使用openpyxl遍历sheet的方法如下:
1. 导入openpyxl模块
```python
import openpyxl
```
2. 打开Excel文件
```python
wb = openpyxl.load_workbook('example.xlsx')
```
3. 获取sheet对象
```python
sheet = wb['Sheet1']
```
4. 遍历sheet中的每一行
```python
for row in sheet.iter_rows():
for cell in row:
print(cell.value)
```
5. 遍历sheet中的每一列
```python
for col in sheet.iter_cols():
for cell in col:
print(cell.value)
```
6. 遍历sheet中的指定范围
```python
for row in sheet.iter_rows(min_row=2, max_row=5, min_col=1, max_col=3):
for cell in row:
print(cell.value)
```
以上就是使用openpyxl遍历sheet的方法。
相关问题
openpyxl遍历sheet
可以使用openpyxl库来遍历Excel文件中的工作表(sheet)。首先,你需要导入openpyxl库并加载Excel文件。然后,你可以通过工作簿对象的`sheetnames`属性获取所有工作表的名称。接下来,你可以使用`get_sheet_by_name`方法选择要遍历的工作表。最后,你可以使用`iter_rows`方法遍历工作表中的每一行,然后使用`iter_cols`方法遍历每一列。下面是一个示例代码:
```python
import openpyxl
# 加载Excel文件
wb = openpyxl.load_workbook('example.xlsx')
# 选择要遍历的工作表
sheet = wb\['Sheet1'\]
# 遍历每一行
for row in sheet.iter_rows():
# 遍历每一列
for cell in row:
print(cell.coordinate, cell.value)
print('--- END OF ROW ---')
```
这段代码会遍历名为'Sheet1'的工作表中的每一行和每一列,并打印出每个单元格的坐标和值。
#### 引用[.reference_title]
- *1* [Python利用openpyxl库遍历Sheet的方法](https://blog.csdn.net/qqyuanhao163/article/details/54976962)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* [Python用openpyxl操作Excel:读写、遍历、单元格读写](https://blog.csdn.net/lilongsy/article/details/127787675)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [openpyxl处理EXCEL](https://blog.csdn.net/qq_41341757/article/details/118966373)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
python openpyxl遍历某一列
可以使用openpyxl库来遍历Excel表格中的某一列。首先,你需要导入openpyxl库并加载Excel文件。然后,选择你要操作的sheet。接下来,你可以使用iter_rows()方法来遍历每一行,然后通过索引来获取某一列的值。下面是一个示例代码:
```python
import openpyxl
# 加载Excel文件
work_book = openpyxl.load_workbook("test.xlsx")
# 选中第一个sheet
work_sheet = work_book\[work_book.sheetnames\[0\]\]
# 遍历某一列
for row in work_sheet.iter_rows():
cell_value = row\[0\].value # 获取第一列的值
print(cell_value)
```
这段代码会遍历Excel表格中的每一行,并打印出第一列的值。你可以根据需要修改索引来获取其他列的值。请确保你已经安装了openpyxl库,可以使用pip install openpyxl命令进行安装。\[3\]
#### 引用[.reference_title]
- *1* [Openpyxl获取指定列](https://blog.csdn.net/cftchaoxiaoshu/article/details/120651965)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down28v1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* [python:openpyxl取某一行、某一列、应用excel函数](https://blog.csdn.net/weixin_66118670/article/details/129883589)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down28v1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [Python用openpyxl操作Excel:读写、遍历、单元格读写](https://blog.csdn.net/lilongsy/article/details/127787675)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down28v1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
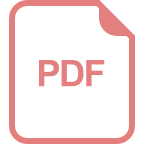
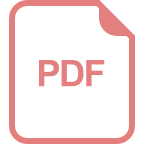












