Java使用ldap API 实现添加数据
时间: 2024-05-16 19:16:13 浏览: 173
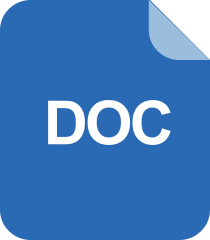
3.3.01_LDAP Java API 使用指南.doc
LDAP(Lightweight Directory Access Protocol)是一种应用层协议,用于访问和维护分布式目录信息服务。在Java中,可以使用JNDI(Java Naming and Directory Interface)API来访问LDAP服务。
下面是使用JNDI API实现LDAP添加数据的示例代码:
```java
import javax.naming.*;
import javax.naming.directory.*;
public class LdapAddDataExample {
public static void main(String[] args) {
// 设置LDAP连接参数
String ldapUrl = "ldap://localhost:389";
String ldapUserDn = "cn=admin,dc=example,dc=com";
String ldapPassword = "password";
String ldapBaseDn = "ou=people,dc=example,dc=com";
// 创建LDAP连接
DirContext ctx = null;
try {
// 初始化环境
Hashtable<String, String> env = new Hashtable<String, String>();
env.put(Context.INITIAL_CONTEXT_FACTORY, "com.sun.jndi.ldap.LdapCtxFactory");
env.put(Context.PROVIDER_URL, ldapUrl);
env.put(Context.SECURITY_AUTHENTICATION, "simple");
env.put(Context.SECURITY_PRINCIPAL, ldapUserDn);
env.put(Context.SECURITY_CREDENTIALS, ldapPassword);
ctx = new InitialDirContext(env);
// 创建LDAP条目
Attributes attrs = new BasicAttributes();
attrs.put("cn", "John Doe");
attrs.put("sn", "Doe");
attrs.put("givenName", "John");
attrs.put("mail", "john.doe@example.com");
attrs.put("userPassword", "password");
Attribute oc = new BasicAttribute("objectClass");
oc.add("inetOrgPerson");
attrs.put(oc);
String entryDn = "cn=John Doe," + ldapBaseDn;
ctx.createSubcontext(entryDn, attrs);
System.out.println("LDAP条目添加成功!");
} catch (NamingException e) {
e.printStackTrace();
} finally {
if (ctx != null) {
try {
ctx.close();
} catch (NamingException e) {
e.printStackTrace();
}
}
}
}
}
```
在上面的代码中,首先设置了LDAP连接参数,然后创建了LDAP连接。接着,创建了LDAP条目的属性,并将其添加到指定的LDAP目录下。
需要注意的是,要使用JNDI API访问LDAP服务,需要在项目中引入javax.naming和javax.naming.directory包。同时,还需要在项目中引入LDAP服务器的JAR包,例如OpenLDAP的JAR包为:openldap.jar。
另外,需要根据实际情况修改代码中的LDAP连接参数和LDAP条目属性。
阅读全文
相关推荐
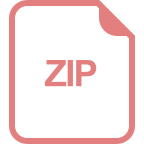
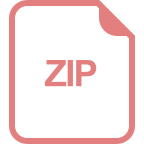















