用JAVA实现LDAP添加数据
时间: 2024-05-01 20:16:09 浏览: 134
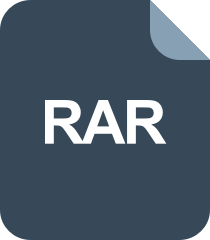
使用Java操作LDAP案例
要使用Java实现LDAP添加数据,需要使用Java提供的LDAP API。以下是一个简单的示例代码,可以将数据添加到LDAP目录中。
```java
import javax.naming.*;
import javax.naming.directory.*;
public class LDAPAddData {
public static void main(String[] args) {
// 定义LDAP服务器的URL和基础DN
String ldapURL = "ldap://ldap.example.com:389";
String baseDN = "dc=example,dc=com";
// 定义LDAP连接的属性
Properties ldapProps = new Properties();
ldapProps.put(Context.INITIAL_CONTEXT_FACTORY, "com.sun.jndi.ldap.LdapCtxFactory");
ldapProps.put(Context.PROVIDER_URL, ldapURL);
ldapProps.put(Context.SECURITY_AUTHENTICATION, "simple");
ldapProps.put(Context.SECURITY_PRINCIPAL, "cn=admin," + baseDN);
ldapProps.put(Context.SECURITY_CREDENTIALS, "password");
try {
// 建立LDAP连接
DirContext ldapContext = new InitialDirContext(ldapProps);
// 创建一个新的LDAP条目
Attributes attrs = new BasicAttributes();
attrs.put("objectClass", "inetOrgPerson");
attrs.put("cn", "John Doe");
attrs.put("sn", "Doe");
attrs.put("givenName", "John");
attrs.put("mail", "johndoe@example.com");
attrs.put("userPassword", "password");
// 将新条目添加到LDAP目录中
Name dn = new CompositeName().add("cn=John Doe");
ldapContext.createSubcontext(dn, attrs);
// 关闭LDAP连接
ldapContext.close();
} catch (NamingException e) {
e.printStackTrace();
}
}
}
```
在上面的示例代码中,我们使用`InitialDirContext`类建立了一个LDAP连接,并通过`BasicAttributes`类创建了一个新的LDAP条目。然后,我们使用`createSubcontext`方法将新条目添加到LDAP目录中。
请注意,上述示例代码中的用户名和密码是明文传输的。在实际生产环境中,应该使用加密传输,并将用户名和密码存储在安全的地方。
阅读全文
相关推荐

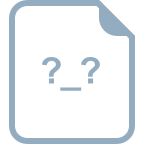
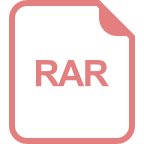
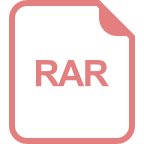

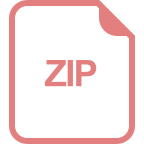
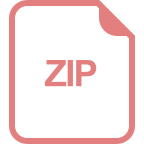
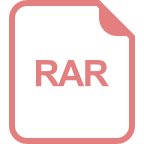
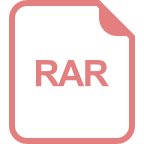
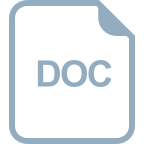
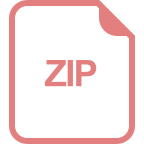
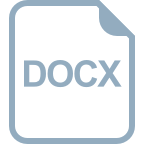
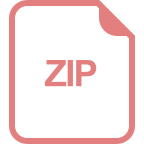
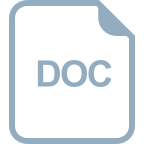
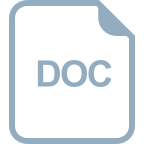
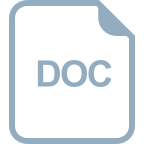