请给出基于c++实现的全景图片拼接的代码
时间: 2023-12-15 14:05:26 浏览: 39
全景图片拼接(Panorama stitching)是一种计算机视觉的应用,它将多张相邻的图片拼接成为一张全景图片。实现全景图片拼接的方法有很多,其中比较经典的方法是通过特征点匹配和图像变换实现。下面给出一个基于OpenCV的C++实现的全景图片拼接的简单代码。
```cpp
#include <opencv2/opencv.hpp>
#include <vector>
using namespace cv;
using namespace std;
int main()
{
// 读入多张图片并存储到向量中
vector<Mat> imgs;
Mat img1 = imread("img1.jpg");
Mat img2 = imread("img2.jpg");
imgs.push_back(img1);
imgs.push_back(img2);
// 特征点检测和描述
Ptr<FeatureDetector> detector = ORB::create();
vector<vector<KeyPoint>> keypoints;
for (int i = 0; i < imgs.size(); i++)
{
vector<KeyPoint> kp;
detector->detect(imgs[i], kp);
keypoints.push_back(kp);
}
Ptr<DescriptorExtractor> extractor = ORB::create();
vector<Mat> descriptors;
for (int i = 0; i < imgs.size(); i++)
{
Mat des;
extractor->compute(imgs[i], keypoints[i], des);
descriptors.push_back(des);
}
// 特征点匹配
Ptr<DescriptorMatcher> matcher = DescriptorMatcher::create("BruteForce-Hamming");
vector<vector<DMatch>> matches;
for (int i = 0; i < imgs.size() - 1; i++)
{
vector<DMatch> match;
matcher->match(descriptors[i], descriptors[i + 1], match);
matches.push_back(match);
}
// 计算变换矩阵
vector<Mat> homographies;
for (int i = 0; i < matches.size(); i++)
{
vector<KeyPoint> kp1 = keypoints[i];
vector<KeyPoint> kp2 = keypoints[i + 1];
vector<Point2f> pts1, pts2;
for (int j = 0; j < matches[i].size(); j++)
{
pts1.push_back(kp1[matches[i][j].queryIdx].pt);
pts2.push_back(kp2[matches[i][j].trainIdx].pt);
}
Mat H = findHomography(pts2, pts1, RANSAC);
homographies.push_back(H);
}
// 拼接图像
Mat result;
for (int i = 0; i < imgs.size(); i++)
{
Mat temp;
warpPerspective(imgs[i], temp, homographies[i-1], Size(2*imgs[i].cols, 2*imgs[i].rows));
if (i == 0)
{
result = temp;
}
else
{
Mat roi(result, Rect(imgs[i].cols/2, 0, imgs[i].cols, imgs[i].rows));
temp(Rect(0, 0, imgs[i].cols, imgs[i].rows)).copyTo(roi);
}
}
// 显示结果
namedWindow("Result", WINDOW_NORMAL);
imshow("Result", result);
waitKey(0);
return 0;
}
```
这段代码实现了以下步骤:
1. 读入多张图片并存储到向量中。
2. 使用ORB算法检测和描述特征点。
3. 使用BruteForce-Hamming算法进行特征点匹配。
4. 使用RANSAC算法计算变换矩阵。
5. 拼接图像。
6. 显示结果。
需要注意的是,在计算变换矩阵时,我们使用了RANSAC算法来剔除错误的匹配点,以提高拼接的准确度。在拼接图像时,我们使用了透视变换(warpPerspective)来将图像进行变换,从而实现拼接。
相关推荐
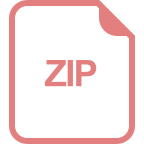
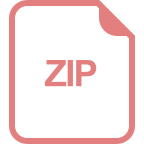














