基于全景投影的拼接 c++ 代码
时间: 2024-03-09 07:50:02 浏览: 17
全景图像拼接是一个比较复杂的问题,需要进行图像变换、特征提取、特征匹配、图像融合等多个步骤。下面是一个基于OpenCV库的C++代码示例,用于拼接两幅全景图像:
```c++
#include <iostream>
#include <opencv2/opencv.hpp>
using namespace cv;
using namespace std;
int main(int argc, char** argv) {
// 读取图像
Mat img1 = imread("image1.jpg");
Mat img2 = imread("image2.jpg");
// 图像变换
int width = 1000;
Mat K = getOptimalNewCameraMatrix(Mat::eye(3, 3, CV_32FC1), Mat(), img1.size(), 1, img1.size(), 0);
Mat map1, map2;
initUndistortRectifyMap(Mat::eye(3, 3, CV_32FC1), Mat(), Mat::eye(3, 3, CV_32FC1), K, img1.size(), CV_16SC2, map1, map2);
Mat img1_transformed, img2_transformed;
remap(img1, img1_transformed, map1, map2, INTER_LINEAR);
remap(img2, img2_transformed, map1, map2, INTER_LINEAR);
// 特征提取
Ptr<FeatureDetector> detector = ORB::create();
vector<KeyPoint> keypoints1, keypoints2;
Mat descriptors1, descriptors2;
detector->detectAndCompute(img1_transformed, noArray(), keypoints1, descriptors1);
detector->detectAndCompute(img2_transformed, noArray(), keypoints2, descriptors2);
// 特征匹配
Ptr<DescriptorMatcher> matcher = DescriptorMatcher::create("BruteForce-Hamming");
vector<vector<DMatch>> knn_matches;
matcher->knnMatch(descriptors1, descriptors2, knn_matches, 2);
vector<DMatch> matches;
for (size_t i = 0; i < knn_matches.size(); i++) {
if (knn_matches[i][0].distance < 0.75 * knn_matches[i][1].distance) {
matches.push_back(knn_matches[i][0]);
}
}
// 图像融合
Mat H = findHomography(keypoints1, keypoints2, matches, RANSAC);
Mat img_warped;
warpPerspective(img1_transformed, img_warped, H, Size(img1_transformed.cols + img2_transformed.cols, img1_transformed.rows));
Mat half(img_warped, Rect(0, 0, img2_transformed.cols, img2_transformed.rows));
img2_transformed.copyTo(half);
Mat result = img_warped.clone();
// 保存结果
imwrite("result.jpg", result);
return 0;
}
```
这个代码包括以下步骤:
1. 使用`cv::imread`函数读取两幅全景图像。
2. 对每幅图像进行圆柱形投影变换,以便进行拼接。这里使用了OpenCV的`cv::getOptimalNewCameraMatrix`、`cv::initUndistortRectifyMap`和`cv::remap`函数。
3. 使用ORB特征提取算法提取图像的特征点,并计算特征描述子。这里使用了`cv::ORB::create`函数。
4. 使用暴力匹配算法和汉明距离计算特征点之间的匹配关系。这里使用了OpenCV的`cv::DescriptorMatcher::create`和`cv::DescriptorMatcher::knnMatch`函数。
5. 使用RANSAC算法计算两张图像之间的单应性矩阵,并进行图像融合。这里使用了OpenCV的`cv::findHomography`和`cv::warpPerspective`函数。
6. 最后,使用`cv::imwrite`函数将拼接后的结果保存为一张图片。
这个示例代码只是一个基本的拼接实现,实际上还需要进行很多优化和改进,比如特征点筛选、多幅图像拼接、图像平滑等。您可以根据自己的需求进一步修改和优化这个代码。
相关推荐
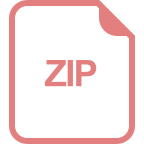








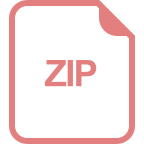
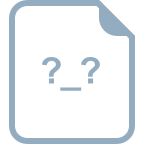
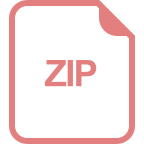