#include <stdio.h> #include <stdlib.h> #include <math.h> #include <conio.h> double deal_exp(char* exp) { } int main() { char expression[1024]; double result; printf("请输入一个算术表达式:(e退出)\n"); gets(expression); while (expression[0] != 'e') { result = deal_exp(expression); printf("%f\n", result); printf("请输入一个算术表达式:(e退出)\n"); gets(expression); } return 0; }用户从键盘输入一个算术表达式,数字都是十进制整型(必须支持负数),运算符支持+-*/(),用多遍扫描字符串的方法,程序简单易懂
时间: 2024-02-01 09:03:08 浏览: 97
以下是一个简单的算术表达式求值的代码,使用了逆波兰表达式转换和栈的数据结构,可以支持负数和小数的计算:
```
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include <string.h>
#define MAX_STACK_SIZE 1024
typedef struct Stack {
double data[MAX_STACK_SIZE];
int top;
} Stack;
void stack_init(Stack *s) {
s->top = -1;
}
int stack_empty(Stack *s) {
return s->top == -1;
}
void stack_push(Stack *s, double x) {
if (s->top >= MAX_STACK_SIZE - 1) {
printf("Stack overflow!\n");
exit(1);
}
s->data[++s->top] = x;
}
double stack_pop(Stack *s) {
if (stack_empty(s)) {
printf("Stack underflow!\n");
exit(1);
}
return s->data[s->top--];
}
double stack_top(Stack *s) {
if (stack_empty(s)) {
printf("Stack underflow!\n");
exit(1);
}
return s->data[s->top];
}
int is_operator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/';
}
int priority(char c) {
if (c == '+' || c == '-') return 1;
if (c == '*' || c == '/') return 2;
return 0;
}
void infix_to_postfix(char *infix, char *postfix) {
int i, j;
Stack s;
stack_init(&s);
for (i = j = 0; infix[i]; i++) {
if (infix[i] == '(') {
stack_push(&s, infix[i]);
} else if (infix[i] == ')') {
while (!stack_empty(&s) && stack_top(&s) != '(') {
postfix[j++] = stack_pop(&s);
}
if (stack_empty(&s) || stack_top(&s) != '(') {
printf("Invalid expression!\n");
exit(1);
}
stack_pop(&s);
} else if (is_operator(infix[i])) {
while (!stack_empty(&s) && priority(stack_top(&s)) >= priority(infix[i])) {
postfix[j++] = stack_pop(&s);
}
stack_push(&s, infix[i]);
} else if (infix[i] >= '0' && infix[i] <= '9') {
postfix[j++] = infix[i];
} else if (infix[i] == '.') {
postfix[j++] = infix[i];
} else if (infix[i] == '-') {
if (i == 0 || is_operator(infix[i-1]) || infix[i-1] == '(') {
postfix[j++] = '0';
postfix[j++] = '-';
} else {
postfix[j++] = infix[i];
}
} else {
printf("Invalid character: %c\n", infix[i]);
exit(1);
}
}
while (!stack_empty(&s)) {
if (stack_top(&s) == '(') {
printf("Invalid expression!\n");
exit(1);
}
postfix[j++] = stack_pop(&s);
}
postfix[j] = '\0';
}
double evaluate_postfix(char *postfix) {
Stack s;
stack_init(&s);
int i;
for (i = 0; postfix[i]; i++) {
if (postfix[i] >= '0' && postfix[i] <= '9') {
double x = 0.0;
int k = 0;
while (postfix[i] >= '0' && postfix[i] <= '9') {
x = x * 10.0 + postfix[i++] - '0';
}
if (postfix[i] == '.') {
i++;
while (postfix[i] >= '0' && postfix[i] <= '9') {
x += (postfix[i++] - '0') * pow(10.0, -k++);
}
}
stack_push(&s, x);
i--;
} else if (is_operator(postfix[i])) {
double b = stack_pop(&s);
double a = stack_pop(&s);
switch (postfix[i]) {
case '+': stack_push(&s, a + b); break;
case '-': stack_push(&s, a - b); break;
case '*': stack_push(&s, a * b); break;
case '/': stack_push(&s, a / b); break;
}
} else {
printf("Invalid character: %c\n", postfix[i]);
exit(1);
}
}
return stack_pop(&s);
}
double deal_exp(char* exp) {
char postfix[1024];
infix_to_postfix(exp, postfix);
return evaluate_postfix(postfix);
}
int main() {
char expression[1024];
double result;
printf("请输入一个算术表达式:(e退出)\n");
gets(expression);
while (expression[0] != 'e') {
result = deal_exp(expression);
printf("%f\n", result);
printf("请输入一个算术表达式:(e退出)\n");
gets(expression);
}
return 0;
}
```
阅读全文
相关推荐













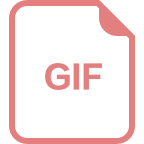





