java数据库5000万数据导出excel表格,给出示例代码
时间: 2023-08-22 22:04:25 浏览: 97
以下是一个基于 Java 和 Apache POI 的示例代码,用于将数据库中的 5000 万条数据导出到 Excel 表格中:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExportToExcel {
public static void main(String[] args) throws SQLException, ClassNotFoundException, IOException {
// 连接数据库
String jdbcUrl = "jdbc:mysql://localhost:3306/myDatabase";
String username = "myUsername";
String password = "myPassword";
Class.forName("com.mysql.jdbc.Driver");
Connection connection = DriverManager.getConnection(jdbcUrl, username, password);
// 执行 SQL 查询
String sql = "SELECT * FROM myTable";
PreparedStatement statement = connection.prepareStatement(sql);
ResultSet resultSet = statement.executeQuery();
// 创建 Excel 工作簿并设置表头
XSSFWorkbook workbook = new XSSFWorkbook();
Row headerRow = workbook.createSheet("Sheet1").createRow(0);
headerRow.createCell(0).setCellValue("Column1");
headerRow.createCell(1).setCellValue("Column2");
// ...
// 将数据写入 Excel 表格
int rowNum = 1;
while (resultSet.next()) {
Row row = workbook.getSheet("Sheet1").createRow(rowNum++);
Cell cell1 = row.createCell(0);
cell1.setCellValue(resultSet.getString("Column1"));
Cell cell2 = row.createCell(1);
cell2.setCellValue(resultSet.getString("Column2"));
// ...
}
// 将 Excel 表格保存到文件
FileOutputStream outputStream = new FileOutputStream("output.xlsx");
workbook.write(outputStream);
workbook.close();
}
}
```
这段代码中使用了 Apache POI 库来操作 Excel 表格。在使用之前需要先下载并添加到项目依赖中。此外,需要根据实际情况修改数据库连接信息和 SQL 查询语句。
阅读全文
相关推荐













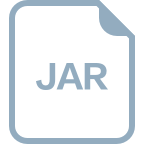


