基于stm32f103c8t6驱动ssd1306oled显示图像代码
时间: 2023-08-01 16:12:36 浏览: 156
以下是基于STM32F103C8T6驱动SSD1306 OLED显示图像的代码:
```c
#include "stm32f10x.h"
#include "stm32f10x_spi.h"
#include "stm32f10x_gpio.h"
#include "stm32f10x_rcc.h"
#define OLED_CS_H() GPIO_SetBits(GPIOB, GPIO_Pin_12)
#define OLED_CS_L() GPIO_ResetBits(GPIOB, GPIO_Pin_12)
#define OLED_DC_H() GPIO_SetBits(GPIOB, GPIO_Pin_10)
#define OLED_DC_L() GPIO_ResetBits(GPIOB, GPIO_Pin_10)
#define OLED_RST_H() GPIO_SetBits(GPIOB, GPIO_Pin_11)
#define OLED_RST_L() GPIO_ResetBits(GPIOB, GPIO_Pin_11)
void SPI_Configuration(void)
{
SPI_InitTypeDef SPI_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_SPI1, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_5 | GPIO_Pin_7;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
SPI_InitStructure.SPI_Direction = SPI_Direction_2Lines_FullDuplex;
SPI_InitStructure.SPI_Mode = SPI_Mode_Master;
SPI_InitStructure.SPI_DataSize = SPI_DataSize_8b;
SPI_InitStructure.SPI_CPOL = SPI_CPOL_High;
SPI_InitStructure.SPI_CPHA = SPI_CPHA_2Edge;
SPI_InitStructure.SPI_NSS = SPI_NSS_Soft;
SPI_InitStructure.SPI_BaudRatePrescaler = SPI_BaudRatePrescaler_4;
SPI_InitStructure.SPI_FirstBit = SPI_FirstBit_MSB;
SPI_Init(SPI1, &SPI_InitStructure);
SPI_Cmd(SPI1, ENABLE);
}
void OLED_Init(void)
{
OLED_RST_L();
delay_ms(1000);
OLED_RST_H();
delay_ms(1000);
OLED_CS_L();
OLED_DC_L();
SPI1_Send(0xAE);
OLED_DC_H();
SPI1_Send(0x40);
SPI1_Send(0xB0);
SPI1_Send(0xC8);
SPI1_Send(0x81);
SPI1_Send(0xff);
SPI1_Send(0xa1);
SPI1_Send(0xa6);
SPI1_Send(0xa8);
SPI1_Send(0x3f);
SPI1_Send(0xd3);
SPI1_Send(0x00);
SPI1_Send(0xd5);
SPI1_Send(0x80);
SPI1_Send(0xd9);
SPI1_Send(0xf1);
SPI1_Send(0xda);
SPI1_Send(0x12);
SPI1_Send(0xdb);
SPI1_Send(0x40);
SPI1_Send(0x20);
SPI1_Send(0x00);
SPI1_Send(0x21);
SPI1_Send(0x00);
SPI1_Send(0x7f);
SPI1_Send(0x22);
SPI1_Send(0x00);
SPI1_Send(0x07);
OLED_DC_L();
SPI1_Send(0xA4);
OLED_DC_H();
SPI1_Send(0xAF);
OLED_CS_H();
}
void OLED_Display_On(void)
{
OLED_CS_L();
OLED_DC_L();
SPI1_Send(0xAE);
OLED_DC_H();
SPI1_Send(0xAF);
OLED_CS_H();
}
void OLED_Display_Off(void)
{
OLED_CS_L();
OLED_DC_L();
SPI1_Send(0xAE);
OLED_CS_H();
}
void OLED_Clear(void)
{
uint8_t i, j;
OLED_CS_L();
for (i = 0; i < 8; i++)
{
OLED_DC_L();
SPI1_Send(0xb0 + i);
SPI1_Send(0x00);
SPI1_Send(0x10);
OLED_DC_H();
for (j = 0; j < 128; j++)
{
SPI1_Send(0);
}
}
OLED_CS_H();
}
void OLED_DrawPixel(uint8_t x, uint8_t y)
{
uint8_t page = y / 8;
uint8_t shift = y % 8;
OLED_CS_L();
OLED_DC_L();
SPI1_Send(0xb0 + page);
SPI1_Send(0x00 + (x & 0x0f));
SPI1_Send(0x10 + ((x >> 4) & 0x0f));
OLED_DC_H();
SPI1_Send(1 << shift);
OLED_CS_H();
}
void OLED_DrawLine(uint8_t x1, uint8_t y1, uint8_t x2, uint8_t y2)
{
uint8_t steep = abs(y2 - y1) > abs(x2 - x1);
uint8_t dx, dy;
int8_t err, ystep;
if (steep)
{
swap(x1, y1);
swap(x2, y2);
}
if (x1 > x2)
{
swap(x1, x2);
swap(y1, y2);
}
dx = x2 - x1;
dy = abs(y2 - y1);
err = dx / 2;
if (y1 < y2)
{
ystep = 1;
}
else
{
ystep = -1;
}
for (; x1 <= x2; x1++)
{
if (steep)
{
OLED_DrawPixel(y1, x1);
}
else
{
OLED_DrawPixel(x1, y1);
}
err -= dy;
if (err < 0)
{
y1 += ystep;
err += dx;
}
}
}
void OLED_DrawRect(uint8_t x1, uint8_t y1, uint8_t x2, uint8_t y2)
{
OLED_DrawLine(x1, y1, x2, y1);
OLED_DrawLine(x1, y1, x1, y2);
OLED_DrawLine(x2, y1, x2, y2);
OLED_DrawLine(x1, y2, x2, y2);
}
void OLED_DrawCircle(int16_t x0, int16_t y0, int16_t r)
{
int16_t x = 0, y = r;
int16_t d = 3 - 2 * r;
while (x <= y)
{
OLED_DrawPixel(x0 + x, y0 + y);
OLED_DrawPixel(x0 + x, y0 - y);
OLED_DrawPixel(x0 - x, y0 + y);
OLED_DrawPixel(x0 - x, y0 - y);
OLED_DrawPixel(x0 + y, y0 + x);
OLED_DrawPixel(x0 + y, y0 - x);
OLED_DrawPixel(x0 - y, y0 + x);
OLED_DrawPixel(x0 - y, y0 - x);
if (d < 0)
{
d += 4 * x + 6;
}
else
{
d += 4 * (x - y) + 10;
y--;
}
x++;
}
}
void OLED_DrawImage(uint8_t *image)
{
uint8_t i, j;
OLED_CS_L();
for (i = 0; i < 8; i++)
{
OLED_DC_L();
SPI1_Send(0xb0 + i);
SPI1_Send(0x00);
SPI1_Send(0x10);
OLED_DC_H();
for (j = 0; j < 128; j++)
{
SPI1_Send(image[i * 128 + j]);
}
}
OLED_CS_H();
}
int main(void)
{
SPI_Configuration();
OLED_Init();
OLED_Display_On();
OLED_Clear();
uint8_t image[1024] = {0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff};
OLED_DrawImage(image);
while (1)
{
}
}
void SPI1_Send(uint8_t data)
{
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_TXE) == RESET);
SPI_I2S_SendData(SPI1, data);
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_RXNE) == RESET);
SPI_I2S_ReceiveData(SPI1);
}
void delay_ms(uint16_t ms)
{
uint32_t i;
for (i = 0; i < ms * 8000; i++);
}
```
需要注意的是,这只是一个简单的例子,实际开发需要根据具体的需求编写更加复杂的程序。同时,需要根据具体的OLED屏幕和STM32微控制器型号,选择相应的驱动程序和通信协议。
阅读全文
相关推荐
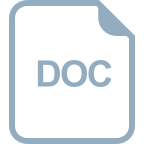


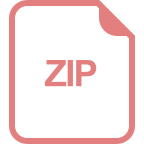
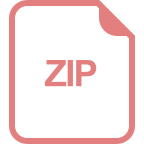
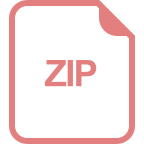




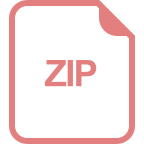
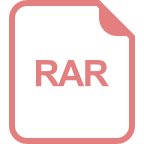
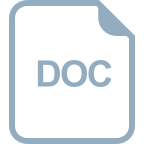

