针对Oracle数据库,Mybatis框架下批量执行多条Update语句
时间: 2024-03-10 14:43:32 浏览: 74
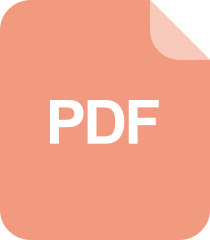
mybatis执行批量更新batch update 的方法(oracle,mysql两种)
针对Oracle数据库,Mybatis框架下批量执行多条Update语句,可以使用Mybatis的BatchExecutor来实现。具体步骤如下:
1. 在Mybatis的配置文件中,将executor-type设置为BATCH,如下所示:
```
<configuration>
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="oracle.jdbc.driver.OracleDriver"/>
<property name="url" value="jdbc:oracle:thin:@localhost:1521:ORCL"/>
<property name="username" value="username"/>
<property name="password" value="password"/>
</dataSource>
<mapper resource="com/example/mapper.xml"/>
<property name="defaultExecutorType" value="BATCH"/>
</environment>
</environments>
</configuration>
```
2. 在Mapper接口中,定义批量执行Update语句的方法,如下所示:
```
public interface UserMapper {
void batchUpdate(List<User> userList);
}
```
3. 在Mapper.xml文件中,编写批量执行Update语句的SQL语句,如下所示:
```
<update id="batchUpdate" parameterType="java.util.List">
<foreach collection="list" item="item" index="index">
update user set name=#{item.name}, age=#{item.age} where id=#{item.id}
</foreach>
</update>
```
4. 在Java代码中,调用Mapper接口的批量执行Update语句的方法,如下所示:
```
List<User> userList = new ArrayList<User>();
// 添加待更新的User对象到userList中
UserMapper userMapper = sqlSession.getMapper(UserMapper.class);
userMapper.batchUpdate(userList);
sqlSession.commit();
```
注意事项:
1. 执行批量更新时,需要使用JDBC事务,因此需要在Mybatis配置文件中配置transactionManager为JDBC。
2. 使用BatchExecutor时,需要手动提交事务,因此需要在Java代码中调用sqlSession.commit()方法提交事务。
3. 执行批量更新时,需要将多条Update语句封装到一个List中,然后传递给Mapper接口的批量执行方法。在Mapper.xml文件中,使用foreach标签遍历List中的每个元素,执行批量更新操作。
阅读全文
相关推荐
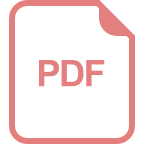
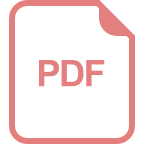















