设计一个循环队列的表示和实现的演示程序,其基本操作有初始化队列,判断队列空否,入队列,出队列等功能,用c语言输出程序代码
时间: 2023-09-01 15:08:39 浏览: 49
好的,下面是循环队列的表示和实现的演示程序,使用C语言编写:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 10
typedef struct {
int data[MAX_SIZE];
int front; // 队头指针
int rear; // 队尾指针
} Queue;
// 初始化队列
void initQueue(Queue *q) {
q->front = q->rear = 0;
}
// 判断队列是否为空
int isEmpty(Queue *q) {
return q->front == q->rear;
}
// 判断队列是否已满
int isFull(Queue *q) {
return (q->rear + 1) % MAX_SIZE == q->front;
}
// 入队列
void enqueue(Queue *q, int x) {
if (isFull(q)) {
printf("Queue is full.\n");
return;
}
q->data[q->rear] = x;
q->rear = (q->rear + 1) % MAX_SIZE;
}
// 出队列
int dequeue(Queue *q) {
if (isEmpty(q)) {
printf("Queue is empty.\n");
return -1;
}
int x = q->data[q->front];
q->front = (q->front + 1) % MAX_SIZE;
return x;
}
int main() {
Queue q;
initQueue(&q);
enqueue(&q, 1);
enqueue(&q, 2);
enqueue(&q, 3);
enqueue(&q, 4);
printf("%d\n", dequeue(&q));
printf("%d\n", dequeue(&q));
enqueue(&q, 5);
enqueue(&q, 6);
printf("%d\n", dequeue(&q));
printf("%d\n", dequeue(&q));
printf("%d\n", dequeue(&q));
printf("%d\n", dequeue(&q));
return 0;
}
```
以上程序中,我们使用结构体定义了一个循环队列,包含了队列元素的数组 `data`,队头指针 `front` 和队尾指针 `rear`。然后,我们实现了队列的基本操作,包括初始化队列、判断队列是否为空、判断队列是否已满、入队列和出队列。最后,我们在 `main` 函数中演示了队列的使用。
相关推荐
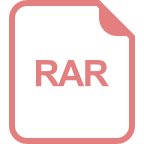
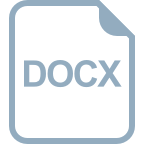














