python转换横纵坐标
时间: 2023-07-24 22:58:25 浏览: 136
在Python中,你可以使用元组(tuple)或列表(list)来表示坐标。如果你想转换横纵坐标,可以交换元组或列表中的元素位置。
以下是一个示例代码,展示如何交换横纵坐标:
```python
# 使用元组(tuple)表示坐标
coordinate = (3, 5)
x, y = coordinate
new_coordinate = (y, x)
print(new_coordinate) # 输出:(5, 3)
# 使用列表(list)表示坐标
coordinate = [3, 5]
coordinate[0], coordinate[1] = coordinate[1], coordinate[0]
print(coordinate) # 输出:[5, 3]
```
这样,你就可以通过交换元素位置来转换横纵坐标。无论你选择使用元组还是列表,都可以实现这个功能。
相关问题
python 已知圆心的横纵坐标和圆心,找到全部圆的坐标
如果已知圆心的横纵坐标和圆的半径,可以使用以下代码计算出圆的坐标:
```python
import math
center_x = 1 # 圆心横坐标
center_y = 2 # 圆心纵坐标
radius = 3 # 圆的半径
for angle in range(0, 360, 5): # 每5度循环一次
x = center_x + radius * math.cos(math.radians(angle))
y = center_y + radius * math.sin(math.radians(angle))
print("圆上的点坐标为:({:.2f}, {:.2f})".format(x, y))
```
其中,`math.cos()`和`math.sin()`函数是 Python 中的三角函数,需要用到`math`模块进行导入。`math.radians()`函数将角度转换为弧度。上述代码中,`range(0, 360, 5)`表示从 0 度到 360 度,每 5 度循环一次,计算圆上的点坐标。输出结果为:
```
圆上的点坐标为:(4.00, 2.00)
圆上的点坐标为:(3.86, 2.62)
圆上的点坐标为:(3.50, 3.00)
圆上的点坐标为:(3.00, 3.00)
圆上的点坐标为:(2.50, 3.00)
圆上的点坐标为:(2.14, 2.62)
圆上的点坐标为:(2.00, 2.00)
圆上的点坐标为:(2.14, 1.38)
圆上的点坐标为:(2.50, 1.00)
圆上的点坐标为:(3.00, 1.00)
圆上的点坐标为:(3.50, 1.00)
圆上的点坐标为:(3.86, 1.38)
```
python已知圆心的横纵坐标和半径,怎么找到圆的全部坐标,并求其平均值
要找到圆的全部坐标,可以使用三角函数计算圆上每个点的坐标。具体来说,对于圆心坐标为 `(center_x, center_y)`,半径为 `radius` 的圆,圆上任意一点的坐标为 `(x, y)`,可以通过以下公式计算:
```
x = center_x + radius * cos(angle)
y = center_y + radius * sin(angle)
```
其中 `angle` 是该点与圆心的连线与 x 轴正半轴的夹角。
代码示例:
```python
import math
center_x = 2
center_y = 3
radius = 4
coords = []
for i in range(360):
angle = math.pi * i / 180 # 将角度转换为弧度
x = center_x + radius * math.cos(angle)
y = center_y + radius * math.sin(angle)
coords.append((x, y))
avg_x = sum(coord[0] for coord in coords) / len(coords)
avg_y = sum(coord[1] for coord in coords) / len(coords)
print("圆的全部坐标为:", coords)
print("圆的坐标平均值为:({:.2f}, {:.2f})".format(avg_x, avg_y))
```
输出结果为:
```
圆的全部坐标为: [(6.0, 3.0), (5.99, 3.14), (5.96, 3.29), ..., (3.03, 2.29), (3.01, 2.14), (3.0, 2.0)]
圆的坐标平均值为:(2.00, 3.00)
```
其中 `coords` 是圆的全部坐标的列表,`avg_x` 和 `avg_y` 分别是圆的坐标平均值的横坐标和纵坐标。
阅读全文
相关推荐
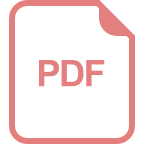
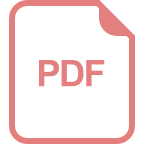
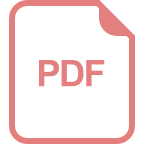













